File Handling in Python
Hey there, budding Pythonista! So, you’re curious about file handling in Python, huh? Well, you’ve come to the right place! In this comprehensive guide, we’re going to break down everything you need to know about file handling in Python, from the basics to more advanced concepts.
What is File Handling in Python?
File handling in Python refers to how Python interacts with files on your computer’s storage system. It allows you to perform various operations such as reading data from files, writing data to files, appending data to existing files, and even deleting files when you’re done with them. In simple terms, file handling lets you work with external data sources seamlessly within your Python programs.
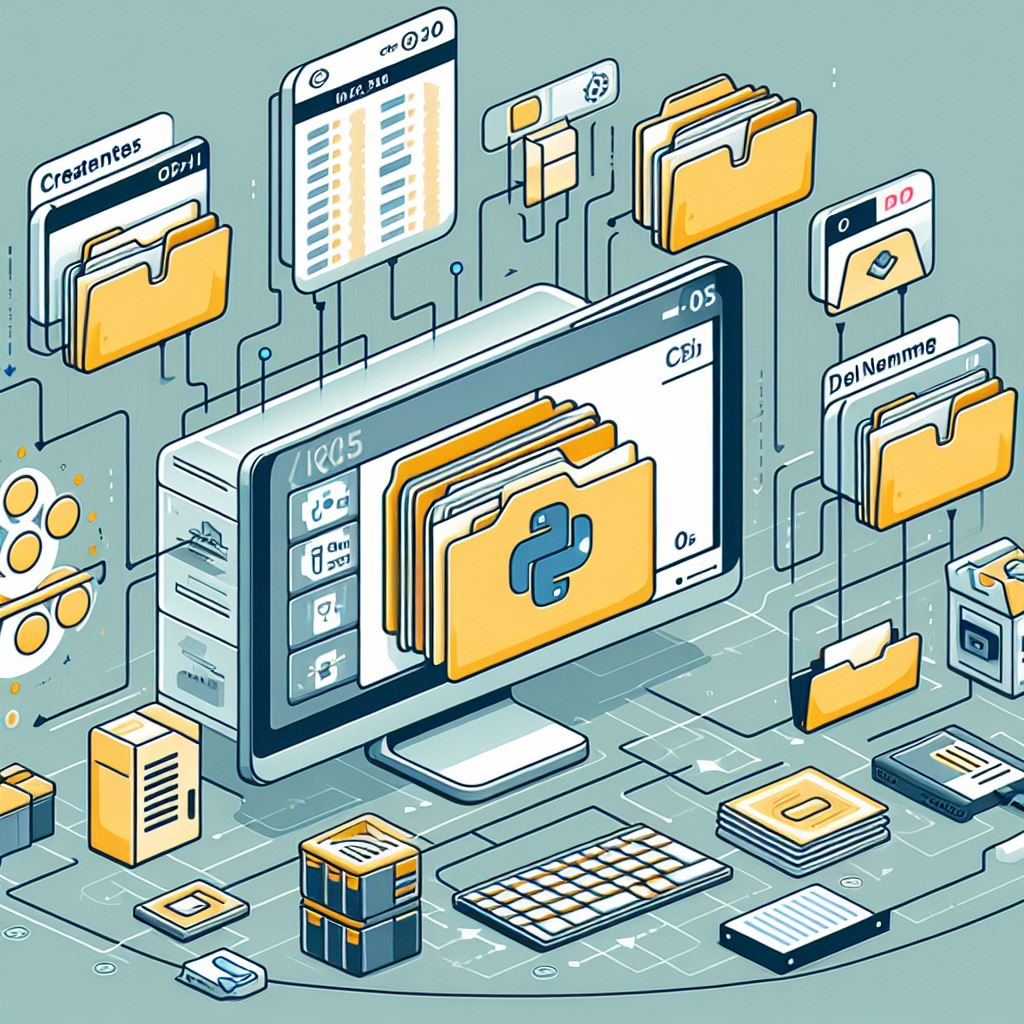
Why is File Handling Important in Python?
Imagine you’re building a chat application, and you need to store chat logs in a file. Or perhaps you’re working on a data analysis project and need to read data from a CSV file. That’s where file handling comes into play! It enables your Python programs to communicate with external files, making it essential for a wide range of applications, from simple scripts to complex software systems.
Types of Files in Python
Before we delve deeper into file handling, let’s understand the different types of files you can work with in Python:
Text Files
Text files are like digital pages where you can write down words, sentences, or any text you want. They’re just like the documents you create in programs like Microsoft Word or Google Docs.
You can use text files for all sorts of things! Maybe you want to keep track of your to-do list, write a story, or save some notes. Text files are perfect for storing plain old text that you can easily read and write.
Binary Files
Binary files are a bit different from text files. Instead of containing readable text, they store data in a special code that computers understand. This could be anything from pictures and music to computer programs and videos.
Binary files are like treasure chests full of digital goodies! You can use them to store all sorts of stuff, like photos from your last vacation, songs from your favorite band, or even the latest video game you downloaded.
CSV Files
CSV stands for Comma-Separated Values. Think of CSV files as tables, like the ones you see in Excel or Google Sheets. Each row represents a different piece of information, and commas are used to separate the different values.
CSV files are super handy for storing data in an organized way. Let’s say you have a list of names and ages that you want to keep track of. You can use a CSV file to store this data neatly, with each person’s name and age in its row.
JSON Files
JSON stands for JavaScript Object Notation. A JSON file stores data in a format that is similar to how objects are written in JavaScript. They’re like little packages containing organized data.
JSON files are great for storing structured data that you want to share between different programs or systems. For example, if you’re building a website and you need to store user information, you can use a JSON file to keep track of usernames, passwords, and other details.
So, there you have it! These are the main types of files you’ll come across when working with Python. Whether you’re writing a simple text document, storing some cool pictures, or managing data for your projects, understanding these file types will help you become a Python pro in no time!
File Handling Basics: Opening and Closing Files in Python
When you want to work with a file in Python, you need to open it first. Think of it like opening a book before you can read or write in it. And just like how you close a book when you’re done reading, it’s important to close a file in Python when you’re done with it.
Opening a File:
To open a file, you use the
function. It’s like turning the key to unlock the door to your file. Here’s how it works:open()
file = open("filename.txt", "mode")
In this code:
is the name of the file you want to open. It’s like telling Python which door to unlock."filename.txt"
is how you want to open the file. There are different modes, like:"mode"
: Read mode. You can only read from the file."r"
: Write mode. You can write to the file, and if it doesn’t exist, Python will create it."w"
: Append mode. You can add new stuff to the end of the file."a"
: Read and write mode. You can both read from and write to the file."r+"
Let’s say you want to open a file named "story.txt"
in write mode:
file = open("story.txt", "w")
This line of code opens the "story.txt"
file in write mode, ready for you to write your content.
Closing a File:
Once you’re done with a file, it’s important to close it properly. This frees up any resources associated with the file and ensures that any changes you’ve made are saved. It’s like closing the door behind you when you leave a room. You don’t want to leave it open, right? Here’s how you close a file in Python:
file.close()
This line of code closes the file
that we opened earlier. It’s like saying, “Okay, we’re done with this file now.”file
Using the with Statement:
Python has a cool feature called the
statement that makes working with files even easier. It’s like having a magic spell that automatically opens and closes the file for you. Here’s how it works:with
with open("story.txt", "r") as file: content = file.read() print(content)
In this example, the
statement opens the with
"story.txt"
file in read mode. Inside the
block, you can do whatever you want with the file. When you’re done, Python automatically closes the file for you, even if an error occurs while working with the file.with
Opening and closing files in Python is like unlocking and locking doors to your digital world. Whether you’re reading from a file, writing to it, or just poking around, knowing how to handle files properly is key to writing awesome Python programs.
How to Work with Files in Python
Now that you have an understanding of file handling basics, let’s explore how to perform various file operations in Python: