Tkinter Message Boxes
Message boxes are vital components of applications, providing a means for effective communication between the application and its users.
Imagine you’re using an application and encounter an error. How would you know what went wrong or what to do next? Message boxes provide a way for applications to communicate important information, warnings, errors, or prompts to users clearly and concisely. They help users understand the state of the application, make informed decisions, and take appropriate actions.
Tkinter includes a dedicated module called messagebox
for creating various types of message boxes in applications. This module offers a range of predefined dialogs, such as information boxes, warning boxes, error boxes, question boxes, and more, making it easy to integrate messaging functionality into Tkinter applications.
To access this module, we just need to import it.
import tkinter as tk from tkinter import messagebox
Mastering Various Message Boxes in Tkinter
Now, let’s take a closer look at the different types of message boxes we can invoke with the messagebox
module.
askokcancel()
This message box is perfect when you need to ask the user for a simple “OK” or “Cancel” decision. It is a way to confirm something.
Arguments:
title
: The title of our message box.message
: What we want to ask the user.
Outcome:
True
if the user clicks “OK”.False
if the user clicks “Cancel”.
Example:
import tkinter as tk from tkinter import messagebox def confirm_action(): result = messagebox.askokcancel("Confirmation", "Do you really want to delete this file?") if result: label.config(text="File deleted.") else: label.config(text="Deletion canceled.") root = tk.Tk() button = tk.Button(root, text="Delete File", command=confirm_action, font=('Arial', 20, 'bold')) button.pack() # Show the result in a label label = tk.Label(root, text="", font=('georgia', 30, 'bold')) label.pack(pady=10) root.mainloop()
Output:

askquestion()
When we need a simple “Yes” or “No” answer, we summon this box. It’s great for straightforward questions.
Arguments:
title
: The title of our box.message
: What we want to ask the user.
Outcome:
"yes"
if the user chooses “Yes”."no"
if the user opts for “No”.
Example:
import tkinter as tk from tkinter import messagebox def ask_preference(): result = messagebox.askquestion("Preference", "Do you like dark mode?") if result == "yes": label.config(text="Dark mode selected.") else: label.config(text="Light mode preferred.") root = tk.Tk() button = tk.Button(root, text="Choose Mode", command=ask_preference, font=('Arial', 20, 'bold')) button.pack() # Show the result in a label label = tk.Label(root, text="", font=('georgia', 30, 'bold')) label.pack(pady=10) root.mainloop()
Output:

askretrycancel()
Ever faced an error and wondered if you should try again? This message box is for those moments.
Arguments:
title
: The title of our box.message
: The message in the box.
Outcome:
True
if the user wants to retry.False
if the user gives up.
Example:
import tkinter as tk from tkinter import messagebox def retry_operation(): result = messagebox.askretrycancel("Retry", "Connection lost. Retry?") if result: label.config(text="Retrying...") else: label.config(text="Operation canceled.") root = tk.Tk() button = tk.Button(root, text="Connect", command=retry_operation, font=('Arial', 20, 'bold')) button.pack() # Show the result in a label label = tk.Label(root, text="", font=('georgia', 30, 'bold')) label.pack(pady=10) root.mainloop()
Output:

askyesno()
Sometimes, we need a “Yes” or “No” without a specific question. This message box works for those scenarios.
Arguments:
title
: The title of our box.message
: What we want to ask.
Outcome:
True
for “Yes”.False
for “No”.
Example:
import tkinter as tk from tkinter import messagebox def confirm_choice(): result = messagebox.askyesno("Confirmation", "Continue with the selected action?") if result: label.config(text="Action confirmed.") else: label.config(text="Action canceled.") root = tk.Tk() button = tk.Button(root, text="Execute", command=confirm_choice, font=('Arial', 20, 'bold')) button.pack() # Show the result in a label label = tk.Label(root, text="", font=('georgia', 30, 'bold')) label.pack(pady=10) root.mainloop()
Output:

askyesnocancel()
Now, imagine a situation where you want to give the user a third option, like “Yes”, “No”, or “Cancel”. This box can handle it.
Arguments:
title
: The title of our box.message
: The message in the box.
Outcome:
True
for “Yes”.False
for “No”.None
for “Cancel”.
Example:
import tkinter as tk from tkinter import messagebox def make_decision(): result = messagebox.askyesnocancel("Decision", "Save changes before exiting?") if result is None: label.config(text="Decision canceled.") elif result: label.config(text="Changes saved.") else: label.config(text="Changes discarded.") root = tk.Tk() button = tk.Button(root, text="Exit", command=make_decision, font=('Arial', 20, 'bold')) button.pack() # Show the result in a label label = tk.Label(root, text="", font=('georgia', 30, 'bold')) label.pack(pady=10) root.mainloop()
Output:

showerror()
When things go wrong, we want to show an error message. This box does that, with an error icon for extra effect.
Arguments:
title
: The title of our message box.message
: The error message.
Example:
import tkinter as tk from tkinter import messagebox def trigger_error(): messagebox.showerror("Error", "An error occurred. Please try again later.") root = tk.Tk() button = tk.Button(root, text="Trigger Error", command=trigger_error, font=('Arial', 20, 'bold')) button.pack() root.mainloop()
Output:

showwarning()
If we need to warn the user about something, this box helps us to do that and it also shows a warning icon.
Arguments:
title
: The title of our message box.message
: The warning message.
Example:
import tkinter as tk from tkinter import messagebox def display_warning(): messagebox.showwarning("Caution", "This action may cause data loss.") root = tk.Tk() button = tk.Button(root, text="Show Warning", command=display_warning, font=('Arial', 20, 'bold')) button.pack() root.mainloop()
Output:

showinfo()
And finally, if we want to share some information with the user, this box pops up with an “OK” button.
Arguments:
title
: The title of our message box.message
: The information we want to share.
Example:
import tkinter as tk from tkinter import messagebox def display_info(): messagebox.showinfo("Information", "Your account has been successfully created.") root = tk.Tk() button = tk.Button(root, text="Show Info", command=display_info, font=('Arial', 20, 'bold')) button.pack() root.mainloop()
Output:
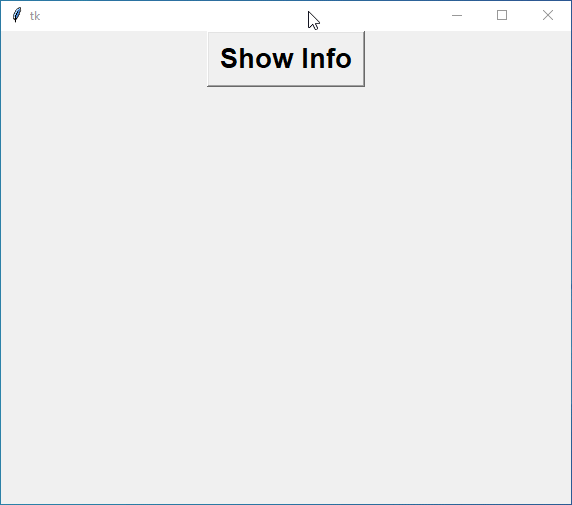