Advanced Looping Techniques in Python
Loops are essential in programming because they automate repetitive tasks and allow code to iterate over collections of data. They save time and effort by executing code multiple times based on conditions or until certain criteria are met.
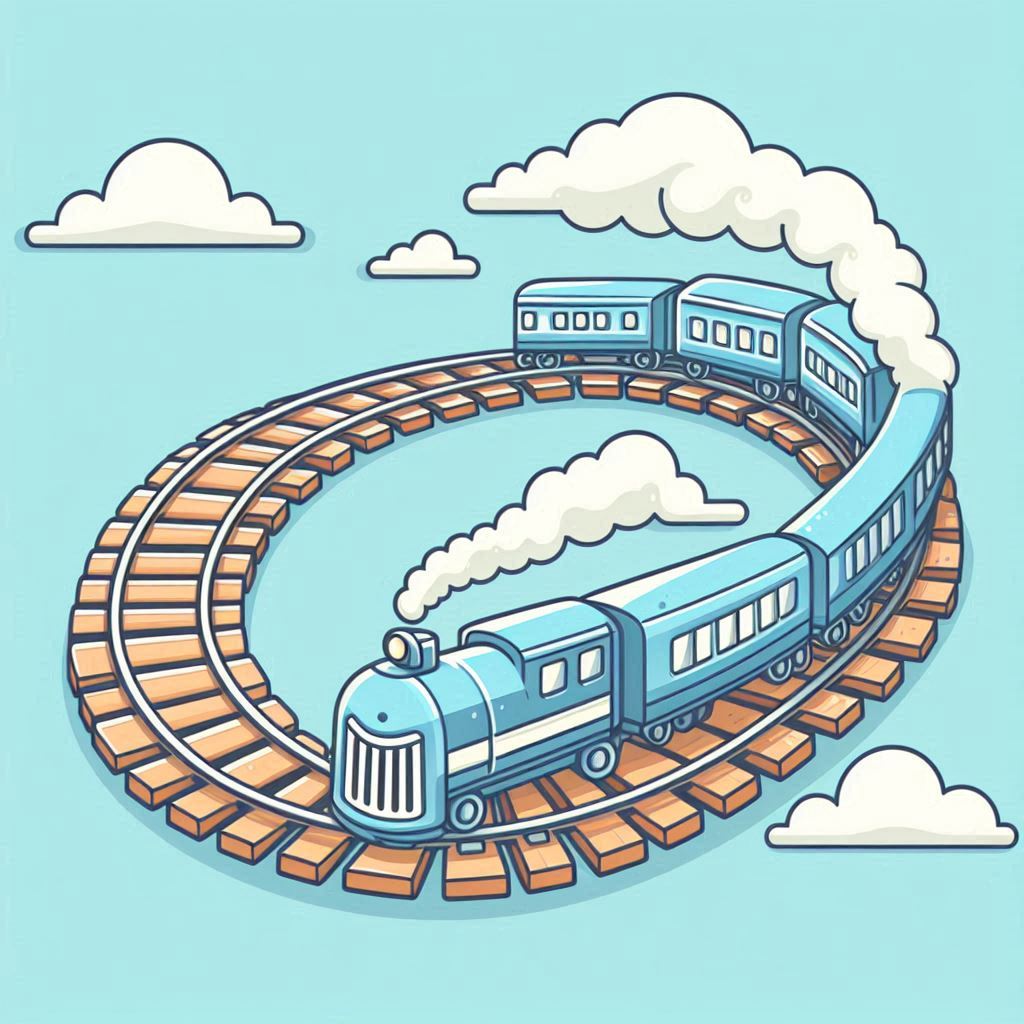
Python provides two main types of loops: for
loops and while
loops. These loops are used in different situations depending on the type of data or specific conditions.
while Loop
The while
loop in Python continuously executes a block of code as long as a specified condition remains true. They are useful when the number of iterations is uncertain or based on a dynamic condition.
Syntax:
while condition: # code to execute while condition is true
Example:
# while loop count = 0 while count < 5: print("Count:", count) count += 1
Output:
Count: 0
Count: 1
Count: 2
Count: 3
Count: 4
Infinite Loops and How to Avoid Them
When the loop condition never becomes false, an infinite loop occurs. To prevent this, ensure your loop condition changes during execution or use break
to exit the loop when needed.
for Loop
The for
loop in Python is used for iterating over sequences like lists, tuples, strings, or ranges. They execute a block of code repeatedly for each item in the sequence.
Syntax:
for element in sequence: # code to execute for each element in the sequence
Example:
# for loop fruits = ["apple", "banana", "orange"] for fruit in fruits: print("Fruit:", fruit)
Output:
Fruit: apple
Fruit: banana
Fruit: orange
Loop Control Statements
Loop control statements help you manage the flow of your loops. Python provides three main control statements: break
, continue
, and pass
. Additionally, loops in Python can have an else
clause.
break Statement
The break
statement lets you exit a loop before it has run all its iterations. When Python hits a break
statement, it stops the loop and jumps to the code after the loop.
Example with while Loop:
# Example of break in a while loop count = 0 while count < 10: if count == 5: break print(count) count += 1
Output:
0
1
2
3
4
Example with for Loop:
# Example of break in a for loop fruits = ["apple", "banana", "cherry", "date"] for fruit in fruits: if fruit == "cherry": break print(fruit)
Output:
apple
banana
continue Statement
The continue
statement skips the rest of the code inside the loop for the current iteration and jumps to the next iteration.
Example with while Loop:
# Example of continue in a while loop count = 0 while count < 5: count += 1 if count == 3: continue print(count)
Output:
1
2
4
5
Example with for Loop:
# Example of continue in a for loop fruits = ["apple", "banana", "cherry", "date"] for fruit in fruits: if fruit == "banana": continue print(fruit)
Output:
apple
cherry
date
pass Statement
The pass
statement is a placeholder that does nothing. It’s useful when you need a statement syntactically but don’t want any code to run.
Example:
numbers = [1, 2, 3, 4, 5] # Example of pass in a loop for number in numbers: if number == 3: pass # Placeholder for future code else: print(number)
Output:
1
2
4
5
The else Clause in Loops
The else
clause in loops runs when the loop completes normally, without encountering a break
statement.
Example:
# Example of else with while loop count = 0 while count < 5: print(count) count += 1 else: print("Loop completed without break")
Output:
0
1
2
3
4
Loop completed without break
Nested Loops
Nested loops are loops within loops. The inner loop runs completely every time the outer loop runs once. This is useful when you need to work with multi-dimensional data, like matrices or tables.
syntax:
for outer_variable in outer_sequence: for inner_variable in inner_sequence: # Code to execute for each pair of outer_variable and inner_variable
Example with Nested while Loops:
# Example of nested while loops i = 1 while i <= 3: j = 1 while j <= 3: print(f"i = {i}, j = {j}") j += 1 i += 1
Output:
i = 1, j = 1
i = 1, j = 2
i = 1, j = 3
i = 2, j = 1
i = 2, j = 2
i = 2, j = 3
i = 3, j = 1
i = 3, j = 2
i = 3, j = 3
Example with Nested for Loops:
# Example of nested for loops fruits = ["apple", "banana", "cherry"] vegetables = ["carrot", "broccoli", "spinach"] for fruit in fruits: for vegetable in vegetables: print(f"Fruit: {fruit}, Vegetable: {vegetable}")
Output:
Fruit: apple, Vegetable: carrot
Fruit: apple, Vegetable: broccoli
Fruit: apple, Vegetable: spinach
Fruit: banana, Vegetable: carrot
Fruit: banana, Vegetable: broccoli
Fruit: banana, Vegetable: spinach
Fruit: cherry, Vegetable: carrot
Fruit: cherry, Vegetable: broccoli
Fruit: cherry, Vegetable: spinach
Looping through Different Data Structures
Python allows you to loop through different types of data structures. Let’s look at some examples.
Example with Tuple:
# Looping through a tuple numbers = (1, 2, 3) for number in numbers: print(number)
Output:
1
2
3
Example with Dictionary:
# Looping through a dictionary student_grades = {"John": "A", "Emma": "B", "Amit": "C"} for student, grade in student_grades.items(): print(f"{student}: {grade}")
Output:
John: A
Emma: B
Amit: C
Example with Set:
# Looping through a set unique_numbers = {1, 2, 3, 4, 5} for number in unique_numbers: print(number)
Output:
1
2
3
4
5
Example with Nested Lists:
# Example with nested lists matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] for row in matrix: for number in row: print(number, end=" ") print()
Output:
1 2 3
4 5 6
7 8 9
Looping with Different Functions
Python has some built-in functions that make loops even more powerful and flexible. Let’s look at how to use the range()
, enumerate()
, and zip()
functions in loops.
Looping with range()
The range()
function creates a sequence of numbers, which is helpful when you need to loop a specific number of times.
Example:
# Looping with range() for i in range(5): print(i)
Output:
0
1
2
3
4
Examples with Different range() Parameters:
print("Starting at 2 and ending before 7") for i in range(2, 7): print(i) print("Starting at 1, ending before 10, stepping by 2") for i in range(1, 10, 2): print(i) print("Counting down from 10 to 1 by 2") for i in range(10, 0, -2): print(i)
Output:
Starting at 2 and ending before 7
2
3
4
5
6
Starting at 1, ending before 10, stepping by 2
1
3
5
7
9
Counting down from 10 to 1 by 2
10
8
6
4
2
Looping with enumerate()
With enumerate()
, you can get both the index and the value in each loop iteration.
Example 1:
# Looping with enumerate() fruits = ["apple", "banana", "cherry"] for index, fruit in enumerate(fruits): print(f"Index: {index}, Fruit: {fruit}")
Output:
Index: 0, Fruit: apple
Index: 1, Fruit: banana
Index: 2, Fruit: cherry
Example 2:
# Starting the index at 1 vegetables = ["carrot", "broccoli", "spinach"] for index, vegetable in enumerate(vegetables, start=1): print(f"Index: {index}, Vegetable: {vegetable}")
Output:
Index: 1, Vegetable: carrot
Index: 2, Vegetable: broccoli
Index: 3, Vegetable: spinach
Looping with zip()
The zip()
function lets you loop through multiple sequences at the same time by pairing up their elements.
Example:
Using zip()
, you can combine and loop through multiple lists at once.
# Looping through three lists fruits = ["apple", "banana", "cherry"] vegetables = ["carrot", "broccoli", "spinach"] prices = [50, 75, 100] for fruit, vegetable, price in zip(fruits, vegetables, prices): print(f"Fruit: {fruit}, Vegetable: {vegetable}, Price: {price}")
Output:
Fruit: apple, Vegetable: carrot, Price: 50
Fruit: banana, Vegetable: broccoli, Price: 75
Fruit: cherry, Vegetable: spinach, Price: 100
Using for Loop for List Comprehensions
A list comprehension is a compact way to generate a list using a single line of code. It consists of brackets containing an expression followed by a for
clause. You can also add if
conditions to filter elements.
Example:
fruits = ["apple", "banana", "cherry"] fruit_lengths = [(fruit, len(fruit)) for fruit in fruits] print(fruit_lengths)
Output:
[('apple', 5), ('banana', 6), ('cherry', 6)]
Using Generator Functions and Expressions with Loops
Generators are a special class of functions that simplify the task of writing iterators. They allow you to iterate through a set of values one at a time without the need to store the entire sequence in memory. This makes them highly efficient for large datasets or streams of data.
Using Loops with Generator Functions
A generator function is defined like a regular function but uses the yield
statement instead of return
to produce a series of values. When yield
is called, the function’s state is saved, and the value is returned to the caller. The next time the generator is called, it resumes execution from where it left off.
Example:
Here’s a simple generator function that yields a sequence of numbers:
fruits = ["apple", "banana", "cherry"] fruit_lengths = [(fruit, len(fruit)) for fruit in fruits] print(fruit_lengths)
You can use this generator in a loop to get values one by one:
gen = number_generator(5) for number in gen: print(number)
Output:
0
1
2
3
4
Generator Expressions
Generator expressions provide a concise way to create generators. They are similar to list comprehensions but use parentheses instead of square brackets. Generator expressions are more memory-efficient because they generate items one at a time.
Example:
Here’s how you can create a generator expression to generate squares of numbers:
squares_gen = (x**2 for x in range(10)) for square in squares_gen: print(square)
Output:
0
1
4
9
16
25
36
49
64
81
Advanced Use Cases
Now let’s see some advanced use cases of generators.
Infinite Sequences
You can create generators for infinite sequences. For example, generating an infinite sequence of Fibonacci numbers:
def fibonacci(): a, b = 0, 1 while True: yield a a, b = b, a + b fib_gen = fibonacci() for _ in range(10): print(next(fib_gen))
Output:
0
1
1
2
3
5
8
13
21
34
Pipeline Processing
Generators can be used in a pipeline to process data through multiple stages. For example, filtering and transforming data:
def filter_even_numbers(numbers): for num in numbers: if num % 2 == 0: yield num def square_numbers(numbers): for num in numbers: yield num**2 numbers = range(10) filtered_gen = filter_even_numbers(numbers) squared_gen = square_numbers(filtered_gen) for num in squared_gen: print(num)
Output:
0
4
16
36
64