Tkinter Label Widget
In Tkinter, a Label widget allows us to display text messages, instructions, or descriptive labels within our GUI applications. This is particularly useful for providing guidance to users or conveying important information.
In addition to text, Labels can also display images, such as icons, logos, or graphical elements, enhancing the visual appeal of the application.
Creating Your First Tkinter Label
Let’s create our first Tkinter Label widget.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() root.title("My Amazing Postcard") # Create the tkinter label label = tk.Label(root, text="Hello, bestie! How are you?", fg="green", font="georgia 20 bold") # Pack the label onto the window using tkinter pack label.pack() # Run the tkinter application root.mainloop()
Output:

Tkinter Label Options
Here are some of the most used options for Tkinter Labels.
text
The text
option simply allows you to specify the text that appears within the Label.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() label = tk.Label(root, text="You're the peanut butter to my jelly!") label.pack() root.mainloop()
Output:
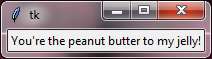
font
With the font
option, you can choose the style, size, and weight of the text in your Label.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() label = tk.Label(root, text="Fancy fonts, here I come!", font=("georgia", 30, "italic")) label.pack() root.mainloop()
Output:

fg and bg
fg
stands for foreground, and bg
stands for background. These options control the color of your text (fg
) and the background color of your Label (bg
).
Example:
import tkinter as tk # Create the main application window root = tk.Tk() label = tk.Label(root, text="I'm feeling blue today!", fg="blue", bg="yellow", font=("georgia", 20, "bold")) label.pack() root.mainloop()
Output:
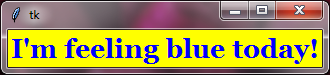
width and height
width
and height
determine the size of your Label. You can specify the dimensions in characters or pixels.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() label = tk.Label(root, text="Just the right size!", width=30, height=6, fg="red", bg="yellow") label.pack() root.mainloop()
Output:
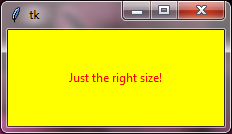
padx and pady
The padding adds space around your Label’s content. padx
is the horizontal padding (left and right), while pady
is the vertical padding (top and bottom).
Example:
import tkinter as tk # Create the main application window root = tk.Tk() label = tk.Label(root, text="Enjoy the extra padding!", padx=40, pady=25, fg="white", bg="green", font=("georgia", 20, "bold")) label.pack() root.mainloop()
Output:

Below are additional standard options available for customization with the Tkinter Label widget:
activebackground
activeforeground
anchor
background
bitmap
borderwidth
compound
cursor
disabledforeground
font
foreground
highlightbackground
highlightcolor
highlightthickness
image
justify
padx
pady
relief
takefocus
text
textvariable
underline
wraplength
To learn more about the options mentioned above, refer to the Tkinter Standard Options
Tkinter Label Methods
Now, let’s explore two of the most commonly used Tkinter label methods: configure()
and cget()
.
configure()
The configure()
method lets you tweak various aspects of a Tkinter label even after creating it. You can change things like the text, font, colors, and more. It’s handy for making dynamic updates to your labels.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() # Create the tkinter label label = tk.Label(root, text="Hello, bestie!") # Pack the label onto the window label.pack() # Update the tkinter label with new text and font label.configure(text="How's it going, pal?", font=("Verdana", 14, "bold")) root.mainloop()
Output:
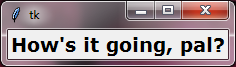
cget
The cget()
method is used to retrieve the current value of any option from your Tkinter label.
Example:
import tkinter as tk # Create the main application window root = tk.Tk() # Create the tkinter label label = tk.Label(root, text="I'm a mysterious label!", font="georgia 18 bold") # Pack the label onto the window label.pack() # Retrieve the current font of the tkinter label current_font = label.cget("font") new_label = tk.Label(root, text=f"Label's current font: {current_font}", font="georgia 18 bold", fg="blue") new_label.pack() root.mainloop()
Output:
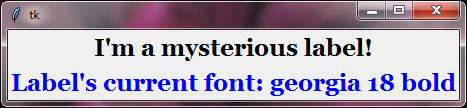