Sets in Python
A set in Python is an unordered collection of unique elements. This means that sets do not allow duplicate values, and the order of elements is not preserved. Sets are used when you want to store multiple items but do not care about the order or the presence of duplicates.
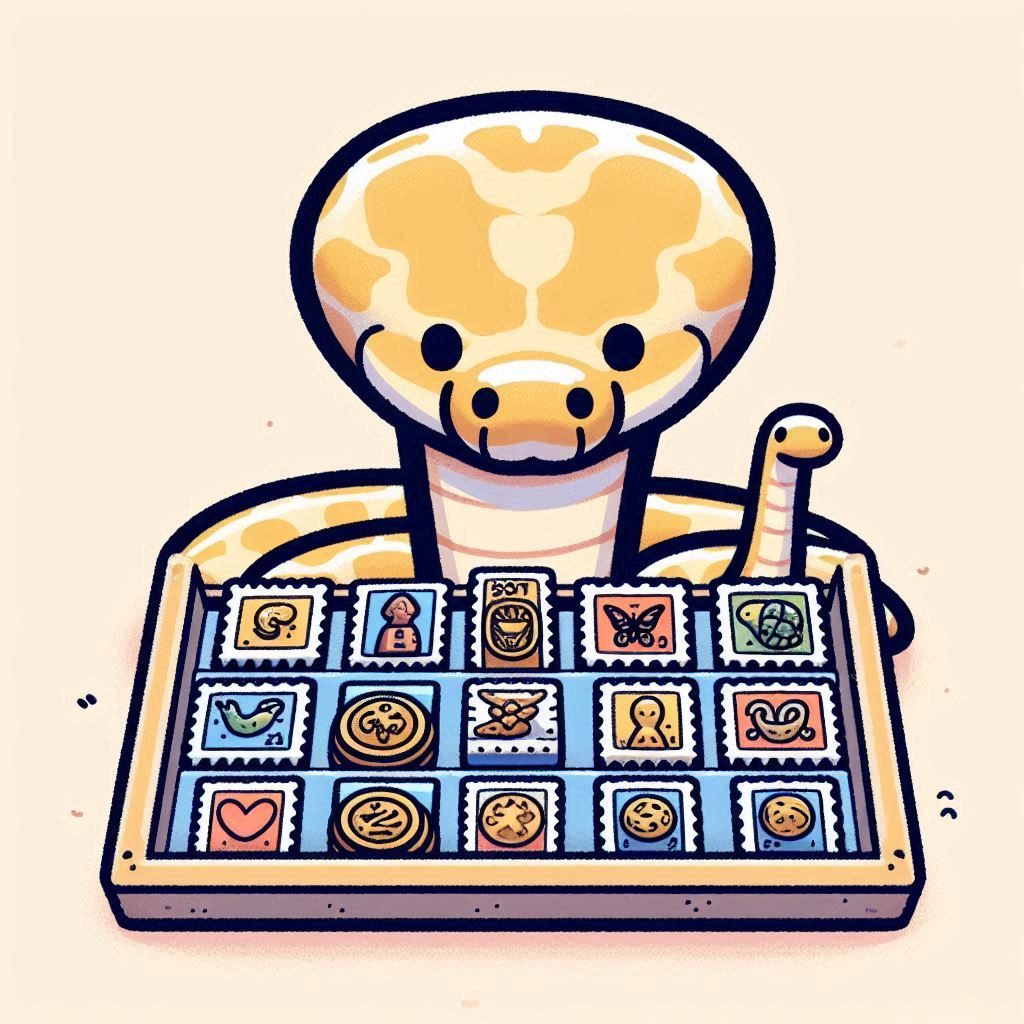
Create Sets in Python
There are two main ways to create a set in Python:
Using Curly Braces {}
You can create a set by placing all the items (elements) inside curly braces {}
, separated by commas.
Example:
# Creating a set using curly braces fruit_set = {"apple", "banana", "cherry"} print(fruit_set)
Output:
{'apple', 'cherry', 'banana'}
Using the set() Function
You can also create a set using the set()
function. This is especially useful when creating a set from an iterable like a list or a string.
Example:
# Creating a set using the set() function numbers_list = [1, 2, 3, 4, 5, 5, 5] numbers_set = set(numbers_list) print(numbers_set)
Output:
{1, 2, 3, 4, 5}
Note: You can use either curly braces or the set()
function to create sets, but note that to create an empty set, you must use the set()
function. Using {}
will create an empty dictionary, not a set.
Example:
# Creating an empty set empty_set = set() print(empty_set) # Using {} will create an empty dictionary, not a set empty_dict = {} print(empty_dict)
Output:
set()
{}
Accessing Set Elements
Sets in Python are unordered collections. This means that the elements in a set do not have a specific order, and their position can change over time. Unlike lists or tuples, you cannot access set elements by their index because sets do not support indexing or slicing.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Attempting to access an element by index will raise an error # fruit_set[0] # This will raise a TypeError
Checking Membership with in Keyword
Although you cannot access elements by index, you can check if an element exists in a set using the in
keyword. This is a common and efficient operation, thanks to the way sets are implemented in Python.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Checking if an element is in the set is_apple_in_set = "apple" in fruit_set print(is_apple_in_set) is_orange_in_set = "orange" in fruit_set print(is_orange_in_set)
Output:
True
False
Using not in Keyword
You can also use the not in
keyword to check if an element is not in the set.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Checking if an element is not in the set is_apple_not_in_set = "apple" not in fruit_set print(is_apple_not_in_set) is_orange_not_in_set = "orange" not in fruit_set print(is_orange_not_in_set)
Output:
False
True
Adding Elements to Sets in Python
Once you’ve created a set, you might want to add new elements to it. Python provides two methods for adding elements to a set: add()
and update()
.
Using the add() Method
The add()
method allows you to add a single element to a set. If the element is already present in the set, the set remains unchanged, as sets do not allow duplicate elements.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Adding a new element fruit_set.add("orange") print(fruit_set) # Trying to add a duplicate element fruit_set.add("banana") print(fruit_set)
Output:
{'orange', 'apple', 'cherry', 'banana'}
{'orange', 'apple', 'cherry', 'banana'}
Using the update() Method
The update()
method allows you to add multiple elements to a set. You can pass any iterable (e.g., list, tuple, string) to the update()
method, and it will add each element of the iterable to the set.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Adding multiple elements using a list fruit_set.update(["mango", "grape"]) print(fruit_set) # Adding multiple elements using a tuple fruit_set.update(("kiwi", "pear")) print(fruit_set) # Adding multiple elements using a string (each character will be added as a separate element) fruit_set.update("berry") print(fruit_set)
Output:
{'grape', 'mango', 'apple', 'cherry', 'banana'}
{'grape', 'mango', 'apple', 'pear', 'kiwi', 'cherry', 'banana'}
{'grape', 'e', 'mango', 'apple', 'pear', 'kiwi', 'cherry', 'y', 'banana', 'b', 'r'}
Removing Elements from Sets in Python
Sets in Python provide several methods to remove elements: remove()
, discard()
, pop()
, and clear()
. Let’s explore these methods one by one.
Using remove() Method
The remove()
method removes a specific element from the set. If the element is not present, it raises a KeyError
.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Removing an element fruit_set.remove("banana") print(fruit_set) # Trying to remove an element that is not in the set raises a KeyError fruit_set.remove("orange") # This will raise a KeyError
Output:
{'apple', 'cherry'}
---------------------------------------------------------------------------
KeyError Traceback (most recent call last)
Cell In[4], line 9
6 print(fruit_set)
8 # Trying to remove an element that is not in the set raises a KeyError
----> 9 fruit_set.remove("orange") # This will raise a KeyError
KeyError: 'orange'
Using discard() Method
The discard()
method also removes a specific element from the set. However, if the element is not present, it does not raise an error.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Discarding an element fruit_set.discard("banana") print(fruit_set) # Discarding an element that is not in the set does not raise an error fruit_set.discard("orange") print(fruit_set)
Output:
{'apple', 'cherry'}
{'apple', 'cherry'}
Using pop() Method
The pop()
method removes and returns an arbitrary element from the set. This is useful when you want to remove elements but do not care which one is removed. If the set is empty, calling pop()
raises a KeyError
.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Popping an element popped_element = fruit_set.pop() print(popped_element) print(fruit_set)
Output:
apple
{'cherry', 'banana'}
Clearing All Elements
The clear()
method removes all elements from the set, resulting in an empty set.
Example:
# Creating a set fruit_set = {"apple", "banana", "cherry"} # Clearing the set fruit_set.clear() print(fruit_set)
Output:
set()
Set Methods in Python
Sets in Python come with a variety of built-in methods that make it easy to perform common operations like union, intersection, and difference. These methods help you to manipulate sets efficiently.
Combining Sets with union()
The union()
method returns a new set containing all elements from the original set and all other sets passed as arguments. Duplicate elements are excluded.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"banana", "kiwi", "mango"} # Using union() union_set = set1.union(set2) print(union_set)
Output:
{'banana', 'mango', 'apple', 'kiwi', 'cherry'}
Finding Common Elements with Intersection Method
The intersection()
method returns a new set containing only the elements that are common to all sets involved.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"banana", "kiwi", "mango"} # Using intersection() intersection_set = set1.intersection(set2) print(intersection_set)
Output:
{3}
Extracting Unique Elements with Difference Method
The difference()
method returns a new set containing elements that are in the original set but not in the other sets passed as arguments.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"banana", "kiwi", "mango"} # Using difference() difference_set = set1.difference(set2) print(difference_set)
Output:
{'apple', 'cherry'}
Unique Elements in Either Set
The symmetric_difference()
method returns a new set containing elements that are in either of the sets but not in both.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"banana", "kiwi", "mango"} # Using symmetric_difference() symmetric_difference_set = set1.symmetric_difference(set2) print(symmetric_difference_set)
Output:
{'mango', 'apple', 'kiwi', 'cherry'}
Checking Subset Relationship
The issubset()
method checks if all elements of the original set are present in the specified set. It returns True
if the original set is a subset, otherwise it returns False
.
Example:
# Creating sets set1 = {"apple", "banana"} set2 = {"apple", "banana", "cherry"} # Using issubset() is_subset = set1.issubset(set2) print(is_subset)
Output:
True
Checking Superset Relationship
The issuperset()
method checks if all elements of the specified set are present in the original set. It returns True
if the original set is a superset, otherwise it returns False
.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"apple", "banana"} # Using issuperset() is_superset = set1.issuperset(set2) print(is_superset)
Output:
True
Checking for Common Elements
The isdisjoint()
method checks if the original set has no elements in common with the specified set. It returns True
if the sets are disjoint, otherwise it returns False
.
Example:
# Creating sets set1 = {"apple", "banana", "cherry"} set2 = {"kiwi", "mango"} # Using isdisjoint() are_disjoint = set1.isdisjoint(set2) print(are_disjoint)
Output:
True
Frozen Sets in Python
A frozen set is an immutable version of a set. This means that once a frozen set is created, its elements cannot be changed, added, or removed. Frozen sets are useful in scenarios where you need a set to be constant and hashable, which allows them to be used as keys in dictionaries or stored in other sets.
To create a frozen set, use the frozenset()
function. You can pass an iterable (like a list, tuple, or set) to this function to initialize the frozen set with its elements.
Example 1:
# Creating a frozen set from a list my_list = [1, 2, 3, 4, 5] frozen_set = frozenset(my_list) print(frozen_set)
Output:
frozenset({1, 2, 3, 4, 5})
Example 2: Using a Frozen Set as a Dictionary Key
# Using a frozen set as a dictionary key my_dict = {frozenset([1, 2, 3]): "Value1", frozenset([4, 5, 6]): "Value2"} print(my_dict)
Output:
{frozenset({1, 2, 3}): 'Value1', frozenset({4, 5, 6}): 'Value2'}
Performing Set Operations
You can perform standard set operations (union, intersection, difference, etc.) on frozen sets, but the results will always be new sets or frozen sets, leaving the original frozen set unchanged.
Example:
# Creating two frozen sets frozen_set1 = frozenset([1, 2, 3]) frozen_set2 = frozenset([3, 4, 5]) # Performing union operation union_set = frozen_set1.union(frozen_set2) print(union_set) # Performing intersection operation intersection_set = frozen_set1.intersection(frozen_set2) print(intersection_set) # Performing difference operation difference_set = frozen_set1.difference(frozen_set2) print(difference_set)
Output:
frozenset({1, 2, 3, 4, 5})
frozenset({3})
frozenset({1, 2})
Looping Through Elements of a Set
Sets are collections of unique elements and do not maintain any order. To iterate through the elements of a set, you can use a for
loop. Here’s how you can do it:
Example:
# Creating a set fruits = {"apple", "banana", "cherry"} # Looping through the set for fruit in fruits: print(fruit)
Output:
apple
cherry
banana
Using enumerate() for Indexed Iteration
While sets do not have a fixed order, you can still use enumerate()
if you need to keep track of the index of each element during iteration. This can be useful in some scenarios.
Example:
# Creating a set fruits = {"apple", "banana", "cherry"} # Looping through the set with index using enumerate() for index, fruit in enumerate(fruits): print(index, fruit)
Output:
0 apple
1 cherry
2 banana
Set Comprehensions
Set comprehensions provide a concise way to create sets. Similar to list comprehensions, set comprehensions allow you to generate sets based on existing iterables while optionally including a condition.
Example:
# Creating a set of even squares even_squares = {x * x for x in range(10) if x % 2 == 0} print(even_squares)
Output:
{0, 64, 4, 36, 16}
Other Set Methods in Python
In addition to basic and common set operations, Python provides several other methods and techniques to work with sets effectively.
Length of a Set
The len()
function returns the number of elements in a set.
Example:
fruits = {'apple', 'banana', 'orange'} print(len(fruits))
Output:
3
Copying a Set
The copy()
method creates a shallow copy of a set. This is useful when you need to duplicate a set without affecting the original set.
Example:
fruits = {'apple', 'banana', 'orange'} fruits_copy = fruits.copy() print(fruits_copy)
Output:
{'orange', 'banana', 'apple'}
You can also create a copy using the set()
constructor:
Example:
# Creating a set original_set = {1, 2, 3} # Copying the set using the set() constructor copied_set = set(original_set) print(copied_set)
Output:
{1, 2, 3}
In-Place Modifications
You can modify sets in place using operators like |=
, &=
, -=
, and ^=
.
Union in Place (|=)
Adds elements from another set.
set1 = {1, 2, 3} set2 = {3, 4, 5} set1 |= set2 print(set1)
Output:
{1, 2, 3, 4, 5}
Intersection in Place (&=)
Retains only elements present in both sets.
set1 = {1, 2, 3} set2 = {2, 3, 4} set1 &= set2 print(set1)
Output:
{2, 3}
Difference in Place (-=)
Removes elements found in another set.
set1 = {1, 2, 3} set2 = {2, 3, 4} set1 -= set2 print(set1)
Output:
{2, 3}
Symmetric Difference in Place (^=)
Keeps elements found in either set but not in both.
set1 = {1, 2, 3} set2 = {2, 3, 4} set1 ^= set2 print(set1)
Output:
{1, 4}
Converting Sets to Other Data Types
If you want to convert your sets into list and tuple, you can use the list()
and tuple()
constructor. You can also convert a set to a dictionary where each element becomes a key with a default value (e.g., None
).
Example:
my_set = {1, 2, 3} my_list = list(my_set) print(my_list) my_tuple = tuple(my_set) print(my_tuple) my_dict = dict.fromkeys(my_set, None) print(my_dict)
Output:
[1, 2, 3]
(1, 2, 3)
{1: None, 2: None, 3: None}