Python Turtle Module Tutorial
The Python Turtle Module is designed to learn programming in an interactive way. It helps you to draw complex graphics and pictures.
Table of Contents
The Turtle module mainly consists of two classes – TurtleScreen Class and RawTurtle Class.
The TurtleScreen Class helps you to create a Screen Object.
The Screen Object is a window or drawing board on which you will draw graphics. The Python Turtle Module provides you with some methods which will help you to change the background color, check the size of the window, and also do some other things that you may want to do.
We can create a Screen Object like this :
import turtle screen=turtle.Screen()
The RawTurtle Class helps you to create a Turtle Object.
The Turtle Object is an arrow shape pen that will help you to draw pictures on the window. The Python Turtle Module provides you functions with the help of which you can move the turtle in different directions to draw pictures and graphics. You can also do many other things with the turtle like change its shape, change the color, etc.
We can create a Turtle Object like this :
import turtle new_turtle=turtle.Turtle()
Basic Turtle Program
import turtle screen=turtle.Screen() new_turtle=turtle.Turtle() turtle.done()
The Turtle module is the built-in module of Python. The first line will import the Turtle module.
The second line will define the Screen Object. Now you will be able to use Screen object functions.
The third line will define a Turtle object. Now you will be able to move this object to draw pictures.
The fourth line will call turtle.done()
function. This function is necessary and it must be the last statement of every turtle program otherwise your program will not work properly.
Output :
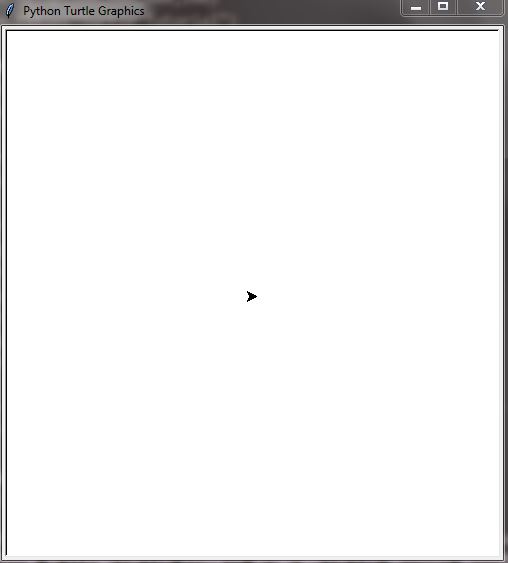
Screen Methods Of Python Turtle Module To Setup The Screen
title(string)
= This function helps you to specify the title of the window.
setup(width, height, startx, starty)
= With this function, you can specify the height and width of the window. You can also specify where you want to place your window on the monitor screen. The startx parameter helps you with horizontal positioning whereas starty will help with vertical positioning. All parameters are optional and should be an integer.
Example :
import turtle screen=turtle.Screen() screen.setup(250,250,400,200) screen.title("Turtle Tutorial") turtle.done()
Output :

window_height()
= This function will return you the current height of the window.
window_width()
= This function returns you the current width of the window.
Example :
import turtle screen=turtle.Screen() screen.setup(300,250,400,200) screen.title("Turtle Tutorial") print(screen.window_height()) print(screen.window_width()) turtle.done()
Output :
250
300
How To Change The Background Of The Screen
bgcolor()
= This function helps you to specify the background color of the window. And if you don’t specify any color, then it will return you the color name or a tuple of three float numbers that define the color of the window.
Example – 1 :
import turtle screen=turtle.Screen() screen.setup(400,400,400,200) screen.title("Turtle Tutorial")) screen.bgcolor('orange') turtle.done()
Output :

Example – 2 :
import turtle screen=turtle.Screen() screen.setup(400,400,400,200) screen.title("Turtle Tutorial")) screen.bgcolor(0.3,0.2,0.6) turtle.done()
Output :
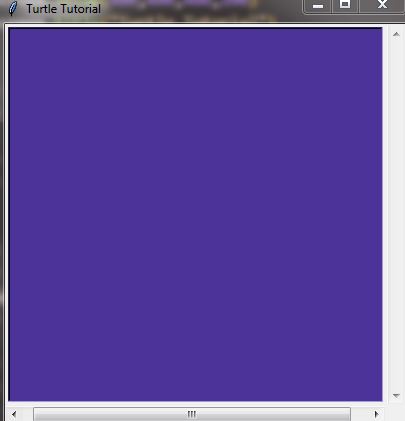
bgpic(picname)
= This function will help you to set the background image of the window. The picname should be a string, gif image name.
Example :
import turtle screen=turtle.Screen() screen.setup(400,400,400,200) screen.title("Turtle Tutorial") screen.bgpic("GB-wp1.gif") turtle.done()
Output :
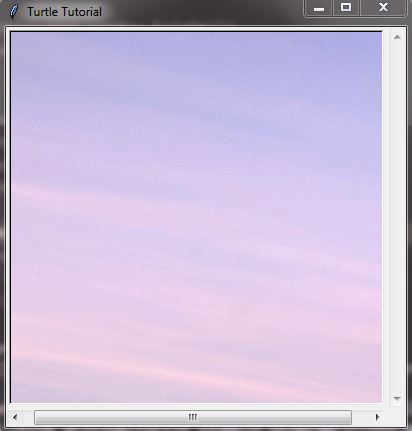
screensize(canvwidth, canvheight, bg)
= This function helps you to specify the width and height of the canvas, but it will not change the size of the window. You can also set the background color of the window by passing the bg argument. But if you pass no arguments, then it will return the current width and height of the canvas.
Example :
import turtle screen=turtle.Screen() screen.title("Turtle Tutorial") screen.bgpic("GB-wp1.gif") screen.screensize(3000,3000,bg='red') turtle.done()
Output :
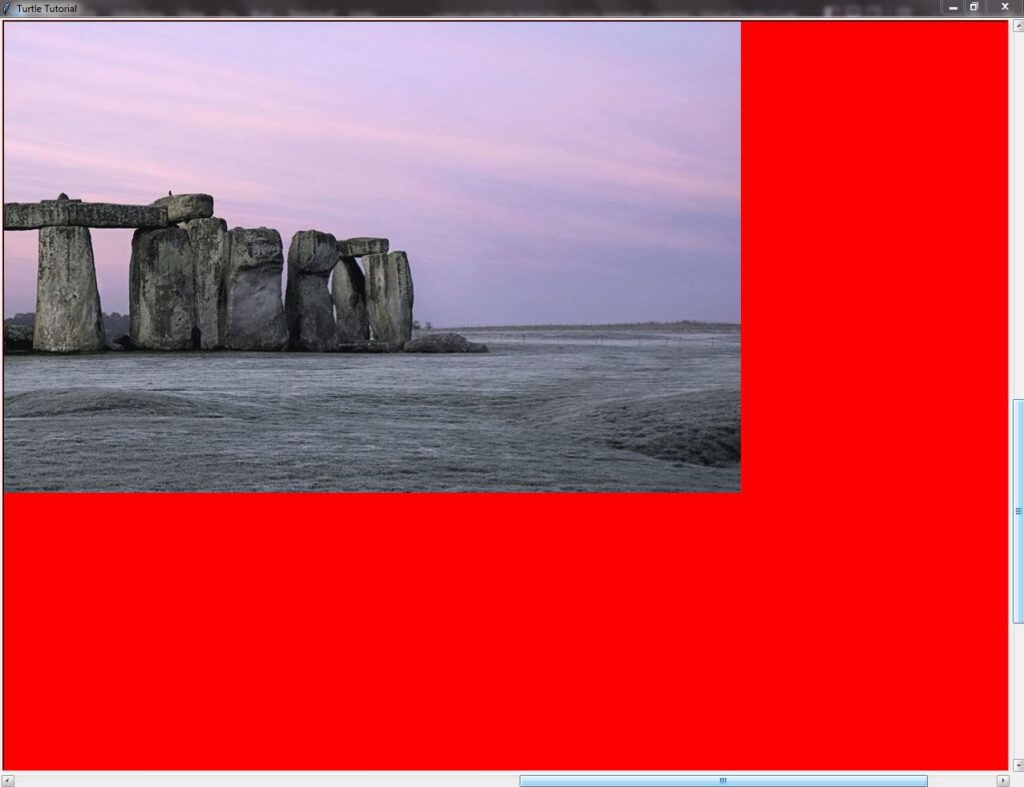
How To Take Text And Numbers As Inputs In Python Turtle Program
textinput(title, prompt)
= It will pop up a dialog box that will take text as input. This function requires two arguments – the title – which will display at the title bar of the dialog box and the prompt – which is the description that will what kind of info is wanted. Both arguments should be a string type.
Example :
import turtle screen=turtle.Screen() screen.setup(400,400,400,200) screen.title("Turtle Tutorial") textinput=screen.textinput("Take text input","What's your name ?") turtle.write(textinput,1,'left') turtle.done()
Output :

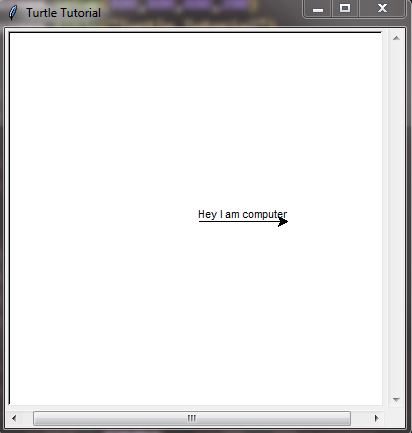
numinput(title, prompt)
= This function also works like the textinput()
but only accepts a number as input.
Example :
import turtle screen=turtle.Screen() screen.setup(400,400,400,200) screen.title("Turtle Tutorial") numinput=screen.numinput("Take number input","Type number") turtle.write(numinput,1,'left') turtle.done()
Output :
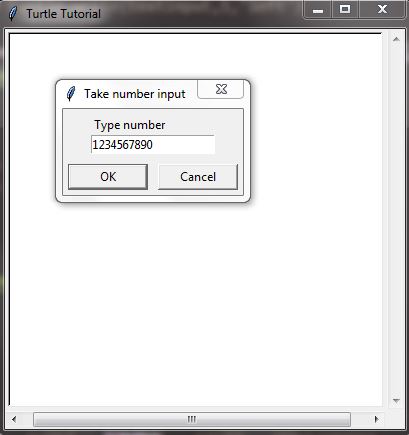
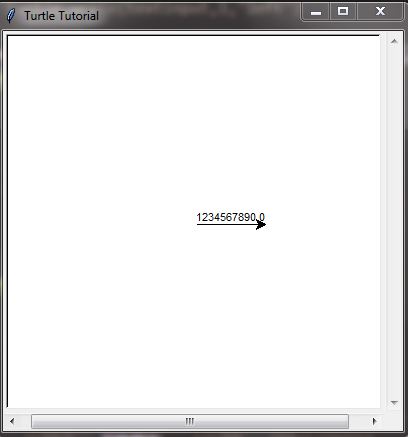
How To Clear And Reset Screen Of Turtle Program
clear()
or clearscreen()
= This function will delete all drawings and turtles from the screen. It also reset the screen to its initial stage where there is no background color or image and only the white screen left.
Example :
import turtle screen=turtle.Screen() new_turtle = turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") new_turtle.forward(200) new_turtle.left(90) new_turtle.forward(200) screen.clear() turtle.done()
Output :
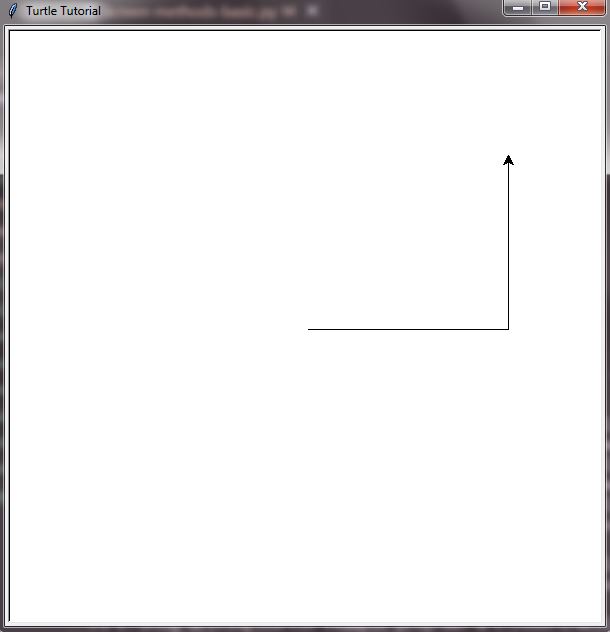
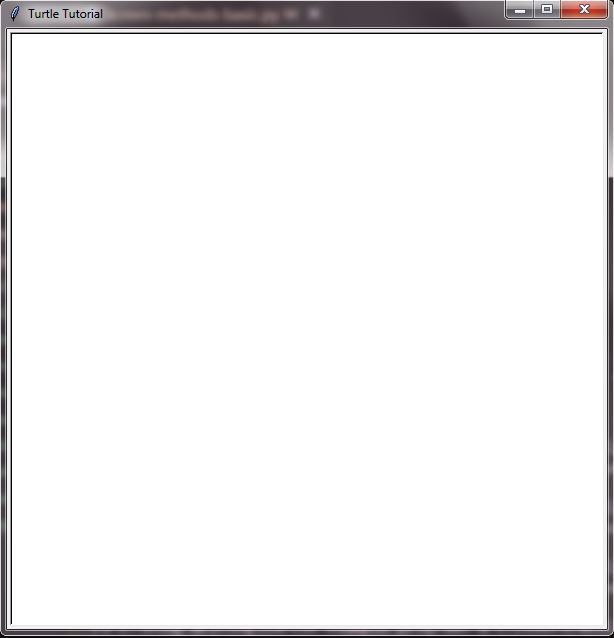
reset()
or resetscreen()
= This function will reset all turtles of the screen to their initial stage.
Example :
import turtle screen=turtle.Screen() new_turtle = turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") new_turtle.forward(200) new_turtle.left(90) new_turtle.forward(200) screen.reset() turtle.done()
Output :
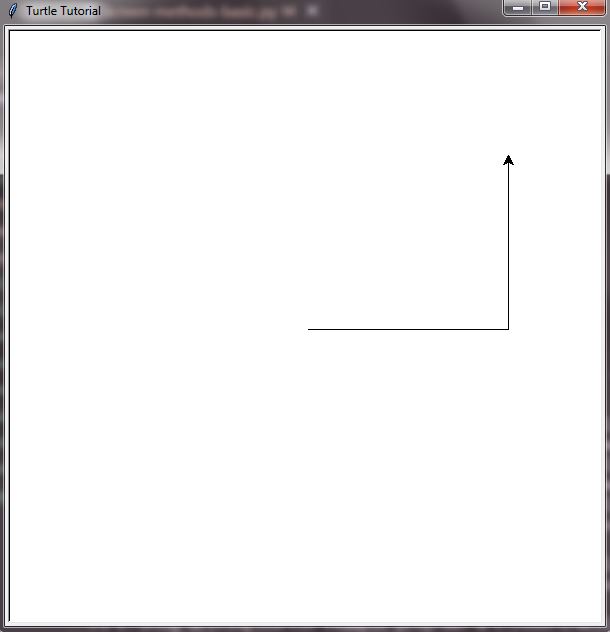
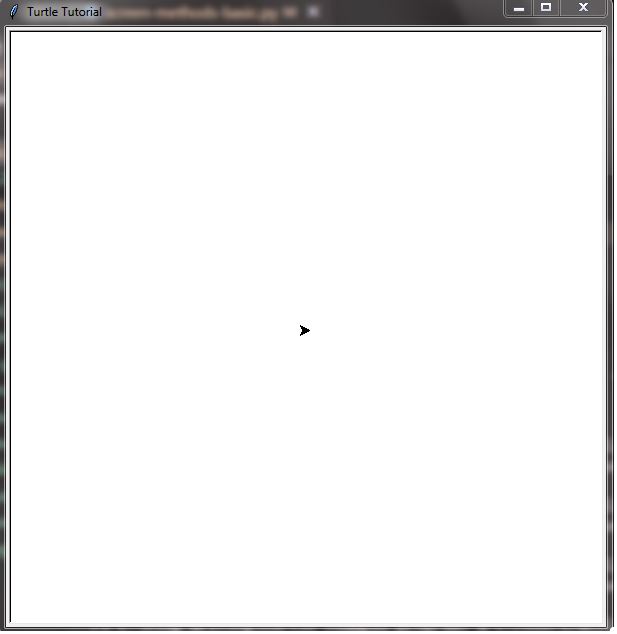
Screen Methods To Close The Turtle Program
exitonclick()
= After using this function, you can close the window by clicking on the screen.
bye()
= This function will close the window.
How To Write Text In Python Turtle Program
The write(arg, move, align, font)
function will help you to write text at the current turtle position on the turtle screen. You can pass four arguments to this function. The arg argument is mandatory while others are optional.
arg | It should be the content you want to write on the turtle screen. |
move | This helps you to decide whether you want to move the turtle while writing on the screen or not. The value should be a boolean. The default value is False. |
align | It specifies the position of the turtle below the text. The value can be – “left”, “right” and “center”. |
font | This will help you to specify the font family, font size, and font style of the text. The value should be a tuple like – (“Arial”, 30, “bold”) |
Example :
import turtle screen=turtle.Screen() new_turtle = turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") new_turtle.write("hey, how are you ?",1,'center', font=("georgia", 20, "italic")) turtle.done()
Output :
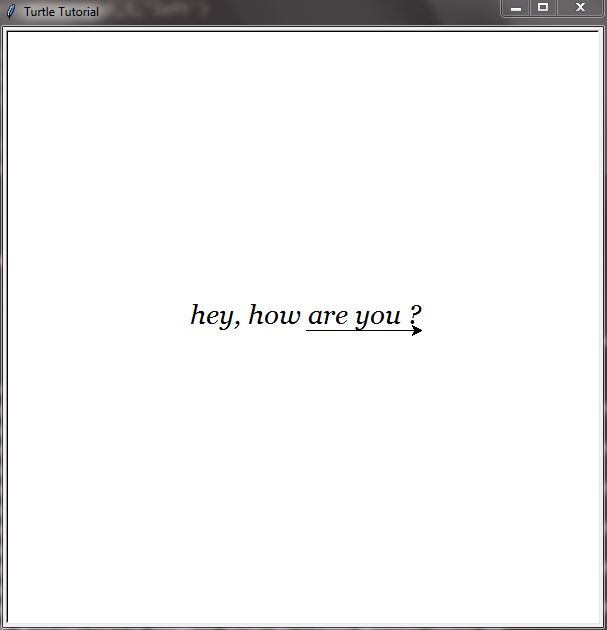
How To Move Turtle And Draw In Python Turtle Program
Following are some functions that will help you to move a turtle and draw something.
forward(distance)
= This function will move the turtle forward by the given distance.
backward(distance)
= It will move the turtle backward by the given distance.
right(angle)
= It turns the turtle right by the given angle.
left(angle)
= It turns the turtle left by the given angle.
Example – 1 :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.forward(200) tt.backward(200) tt.forward(100) tt.right(90) tt.forward(100) tt.right(90) tt.forward(100) tt.right(90) tt.forward(200) turtle.done()
Output :
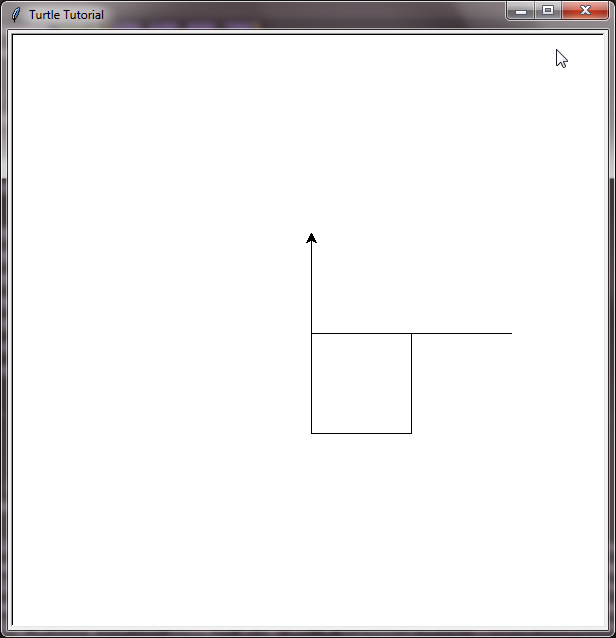
Example – 2 :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") for i in range(0,8): tt.forward(80) tt.right(45) turtle.done()
Output :
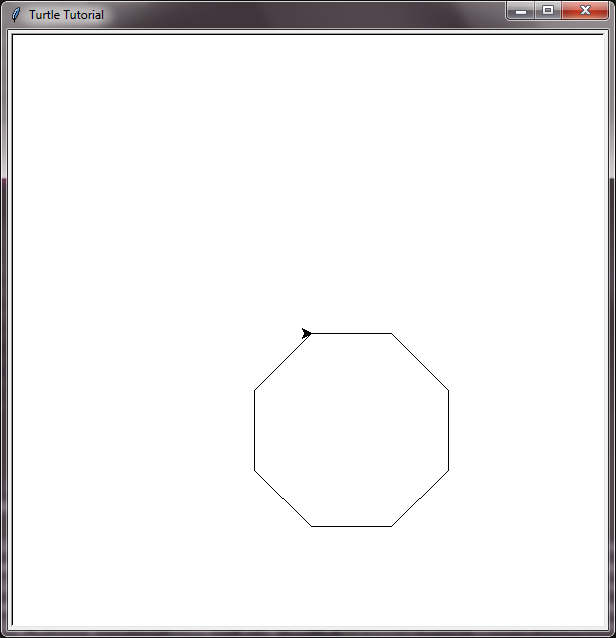
setx(x)
= It will set the x-coordinate of the turtle to the given x.
sety(y)
= It will set the y-coordinate of the turtle to the given y.
xcor()
= It returns the current x-coordinate of the turtle.
ycor()
= It returns the current y-coordinate of the turtle.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.setx(200) tt.write((tt.xcor(), tt.ycor())) tt.sety(200) tt.write((tt.xcor(), tt.ycor())) tt.setx(0) tt.write((tt.xcor(), tt.ycor())) tt.sety(0) tt.write((tt.xcor(), tt.ycor())) turtle.done()
Output :
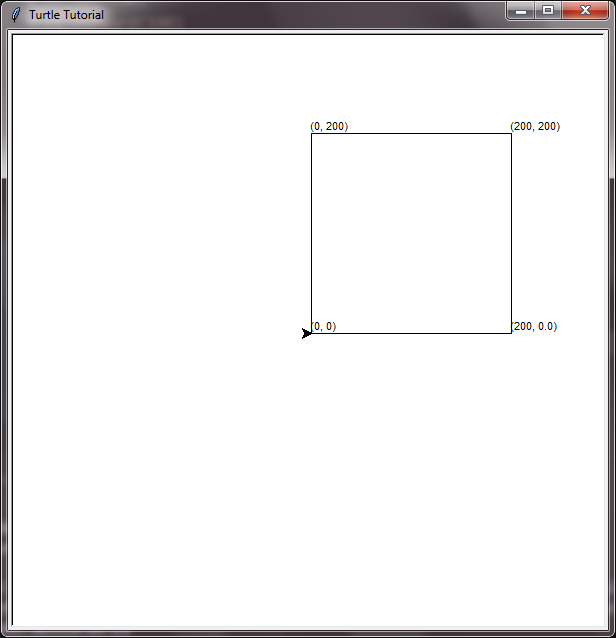
setpos(x,y)
or goto(x,y)
or setposition(x,y)
= These functions will move the turtle without changing its orientation to the absolute position. You have to pass specific x-coordinate and y-coordinate as the arguments of these functions.
position()
= This function returns a tuple of current values of the x-coordinate and y-coordinate of the turtle that is telling the location of the turtle.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.setpos(100,100) tt.write(tt.position()) tt.goto(-100,100) tt.write(tt.position()) tt.setposition(0,0) tt.write(tt.position()) turtle.done()
Output :
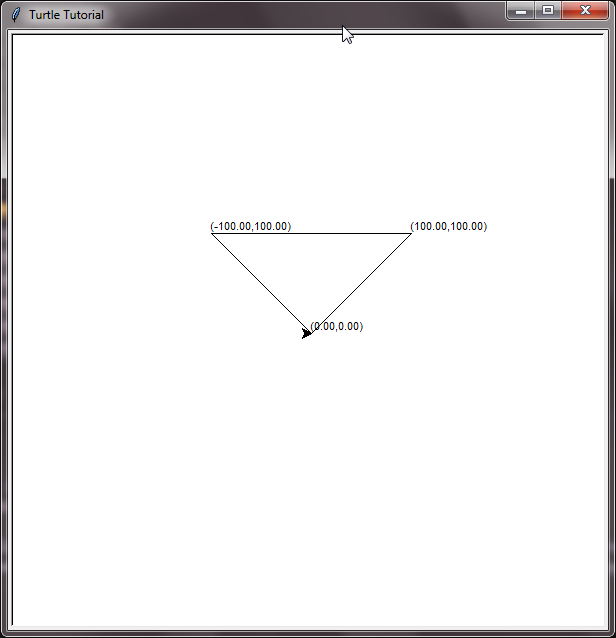
seth(angle)
or setheading(angle)
= These functions help you to change the orientation of the turtle. You have to pass angle as an argument.
heading()
= It returns you an angle that defines the current orientation of the turtle.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.setheading(45) tt.write(tt.heading()) tt.forward(200) tt.seth(180) tt.write(tt.heading()) tt.forward(200) turtle.done()
Output :
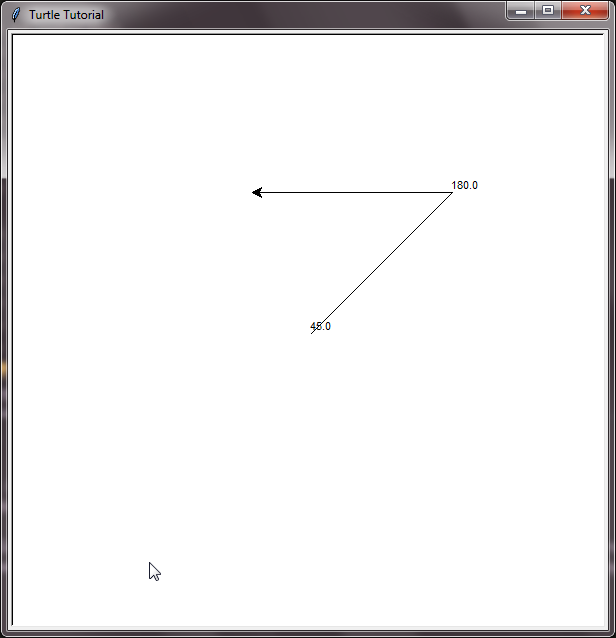
home()
= This function will move the turtle to its origin and also change the turtle orientation to its start orientation.
How To Create Dots And Stamps In Python Turtle Program
dot(size, color)
= It will draw a dot at the current turtle position with the given size. You can also specify the color of the dot by passing a color name as an argument.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.goto(100,100) tt.dot(50,'red') tt.goto(0,200) tt.dot(50,'green') tt.home() tt.dot(50,'blue') turtle.done()
Output :
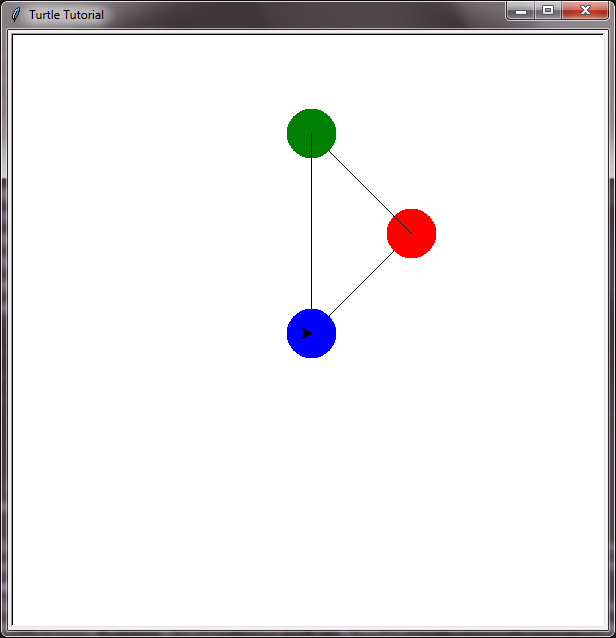
stamp()
= This function will stamp a copy of the turtle at the current turtle position and returns you a stamp_id
that you can use with the clearstamp()
function.
clearstamp(stamp_id)
= This function will delete the stamp of the given stamp_id.
clearstamps()
= It will delete all the stamps from the turtle screen.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.goto(100,100) first_stamp=tt.stamp() tt.goto(0,200) second_stamp=tt.stamp() tt.home() tt.clearstamp(first_stamp) tt.clearstamps() turtle.done()
Output :
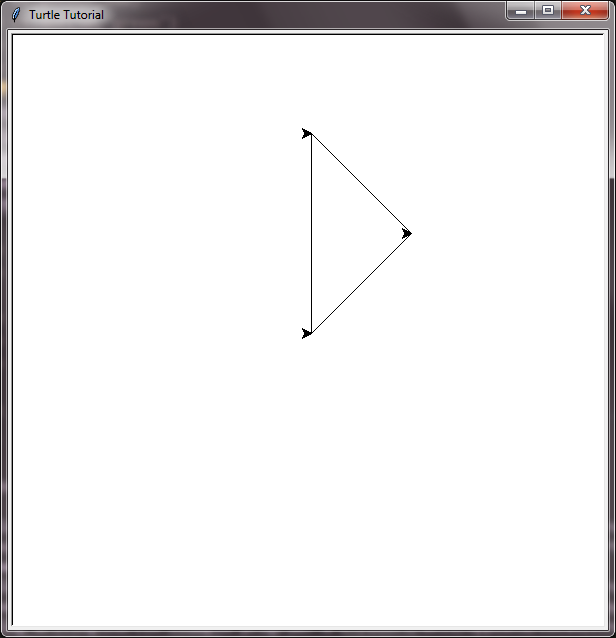
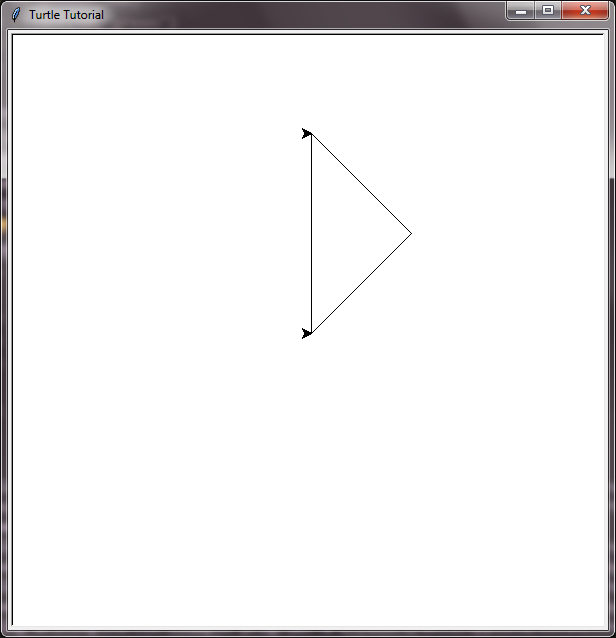
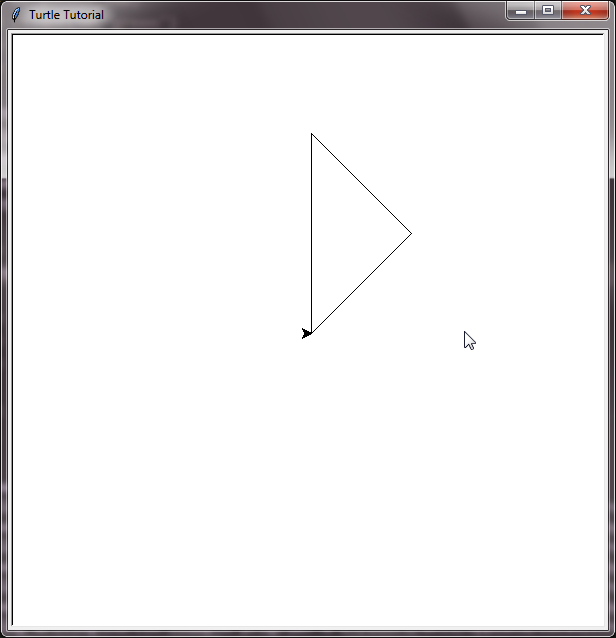
How To Create Circles In Python Turtle Program
circle(radius, extent, steps)
= With the help of this function, you can draw a circle on the turtle. You can pass three arguments to this function.
radius | It determines the size of the circle. |
extent | It decides which part of the circle should be drawn. For example, if specify the extent to 180, then the function draws a half circle. |
step | This helps you to specify how many steps should take to draw the circle. |
The radius argument is necessary while the other two are optional.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.goto(100,100) first_stamp=tt.stamp() tt.goto(0,200) second_stamp=tt.stamp() tt.home() tt.clearstamp(first_stamp) tt.clearstamps() turtle.done()
Output :
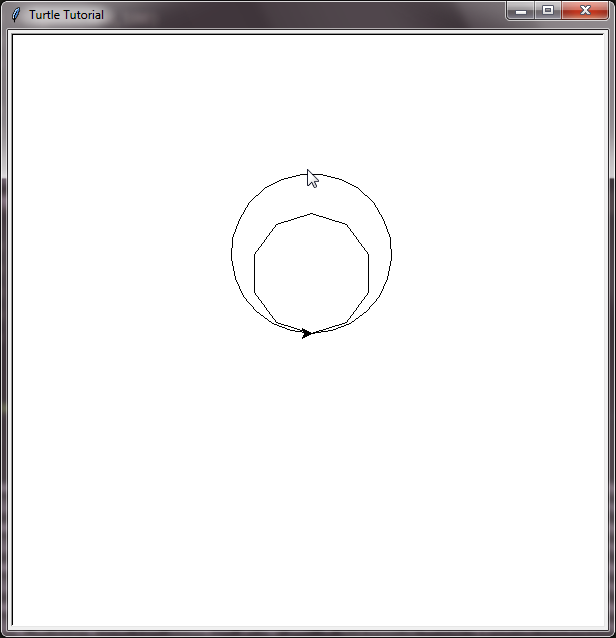
Some Other Important Methods Of Python Turtle Module For Turtle
undo()
= This function will undo the previous turtle action.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt.goto(100,100) tt.goto(0,200) tt.home() for i in range(4): tt.undo() turtle.done()
distance(x,y)
= This function will tell you the distance to the position defined by given x and y from the current turtle position. With this function, you can also get the distance between two turtles.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(600,600,400,200) screen.title("Turtle Tutorial") tt1=turtle.Turtle() tt1.setpos(100,100) tt2=turtle.Turtle() tt2.setpos(-100,-100) tt.write(tt1.distance(tt2)) turtle.done()
Output :
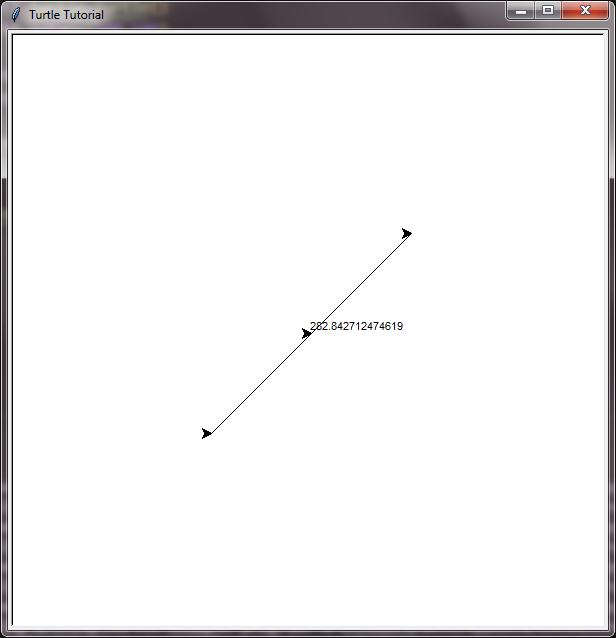
speed(speed)
= With this function, you can specify the speed of the turtle. The value should be an integer(0 to 10) or a speed string.
Following are the speed strings and their corresponding integer values that you can use as parameters.
“fastest” | 0 |
“fast” | 10 |
“normal” | 6 |
“slow” | 3 |
“slowest” | 1 |
You can use this function like this :
tt=turtle.Turtle() tt.speed('slowest')
hideturtle()
= This function will hide the turtle so that when the turtle moves, you can only see lines drawn by the turtle.
You can use this function like this :
tt.hideturtle()
showturtle()
= If the turtle is hidden, then you can use this function to make the turtle visible again.
You can use this function like this :
tt.showturtle()
Pen Methods Of Python Turtle Module
penup()
= After calling this function, you can move the turtle but it will not draw any lines.
pendown()
= If the pen is up, then you can call this function to again down the pen. After that, the turtle will draw lines while moving.
pensize(width)
= This function will help you to specify the width of the lines drawn while moving the turtle.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.pensize(20) tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) turtle.done()
Output :
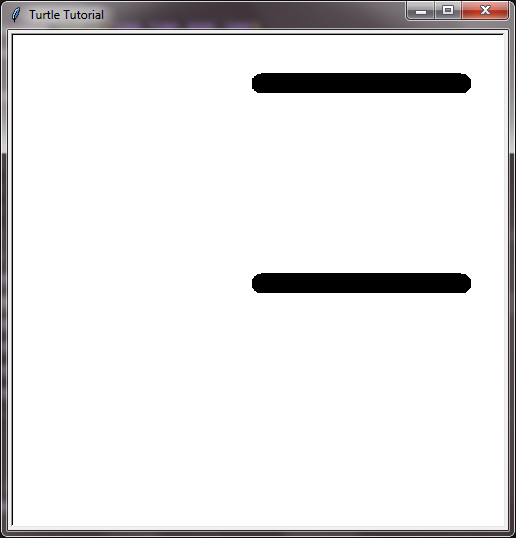
pencolor(color)
= With this function, you can set the color of the lines. You can pass a color name or a rgb color-tuple as an argument to this function.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.pencolor("yellow") tt.hideturtle() tt.pensize(20) tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) turtle.done()
Output :
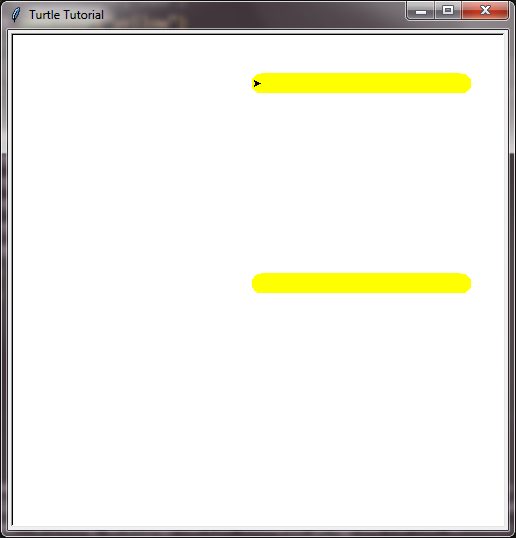
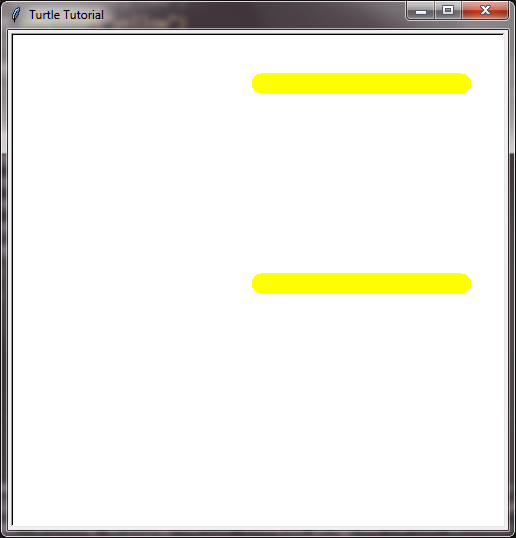
How To Fill Color Inside The Drawn Shape
You have to call the following two turtle functions to fill the color inside the shape.
begin_fill()
= You have to call this function before starting to draw the shape that you want to fill.
end_fill()
= This function will fill the shape that you draw after calling the begin_fill()
function. You should call this function after completing the drawing.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.pencolor("yellow") tt.begin_fill() tt.pensize(20) tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) tt.end_fill() turtle.done()
Output :
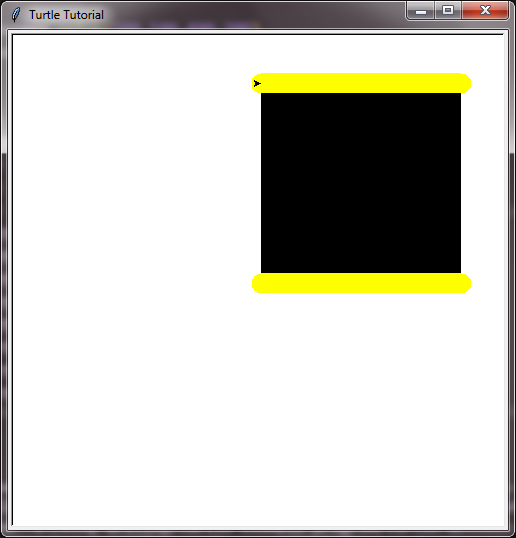
fill_color(color)
= This function will help you to specify the color that you want to fill inside the shape. You can pass a color name or a rgb color-tuple as an argument to this function. You should call this function before calling begin_fill()
.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.pencolor("yellow") tt.fillcolor("red") tt.begin_fill() tt.pensize(20) tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) tt.end_fill() turtle.done()
Output :

Short-Hand Methods For Pen
color(color1, color2)
= This function helps you to specify the pencolor and fillcolor both at a time. The color1 will use as a pencolor while color2 will use as a fillcolor. If you do not pass any argument, then it returns a tuple of the current pencolor and current fillcolor.
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.color('red','yellow') tt.begin_fill() tt.pensize(20) tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) tt.end_fill() turtle.done()
Output :
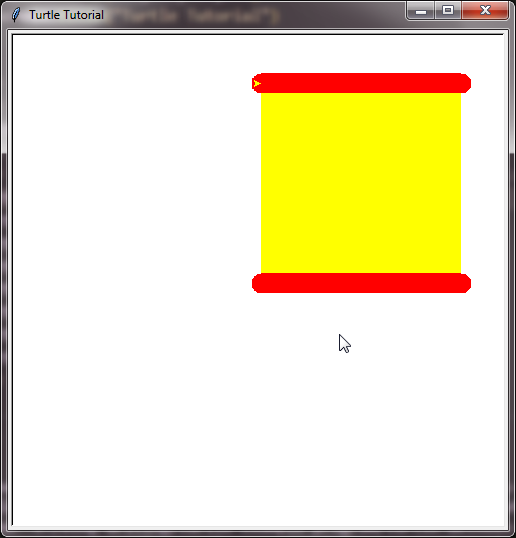
pen(shown, pencolor, fillcolor, pensize, speed)
= This function is the short-hand function that will help you to specify the values of all pen attributes at a time.
Following is the list of option-value pairs that you can use with this function.
shown | True/False or 0 or 1. It decides whether you want to hide the turtle or not. |
pencolor | a color name or a rgb color-tuple |
fillcolor | a color name or a rgb color-tuple |
pensize | positive number |
speed | an integer (0 to 10) |
Example :
import turtle screen=turtle.Screen() tt=turtle.Turtle() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt.pen(shown=0,pencolor='orange',fillcolor='purple',pensize=30, speed=1) tt.begin_fill() tt.setx(200) tt.penup() tt.sety(200) tt.pendown() tt.setx(0) tt.end_fill() turtle.done()
Output :
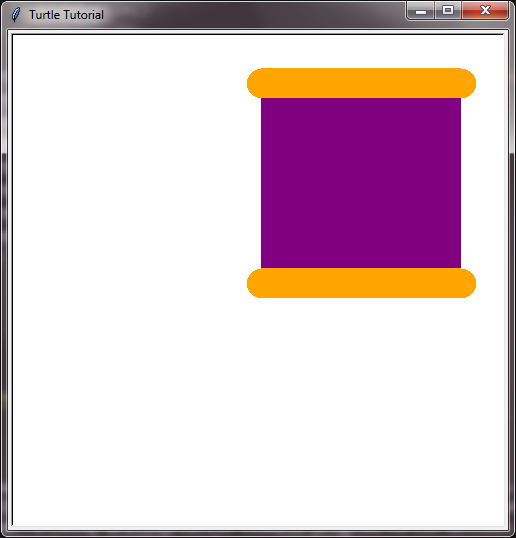
Events In Python Turtle Module
We can bind both screen and turtle with the functions which will be invoked when the mouse and keyboard events occur.
Functions For Mouse Events On The Turtle
onclick(func, btn, add)
= This function will invoke a given func, when the user clicks on the turtle. The func will take the x-coordinate and y-coordinate of the clicked point as the parameters.
Along with func argument, you can also pass two other arguments to this function.
The btn – determines the mouse button, by default value is 1(left-mouse-button), and the other parameter add – decides whether you can bind multiple functions or not, the value should be a boolean.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() tt.speed(0) def func(x,y): tt.right(x*10) tt.forward(y*5) tt.onclick(func) turtle.done()
Output :
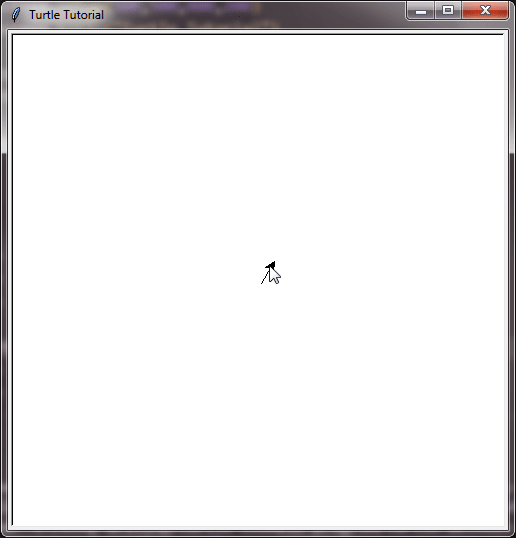
onrelease(func, btn, add)
= All parameters will get the same as the onclick()
. But this time, the mouse button release on the turtle will invoke the func function.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() tt.speed(3) def func(x,y): tt.right(x*10) tt.forward(y*5) tt.onrelease(func) turtle.done()
Output :
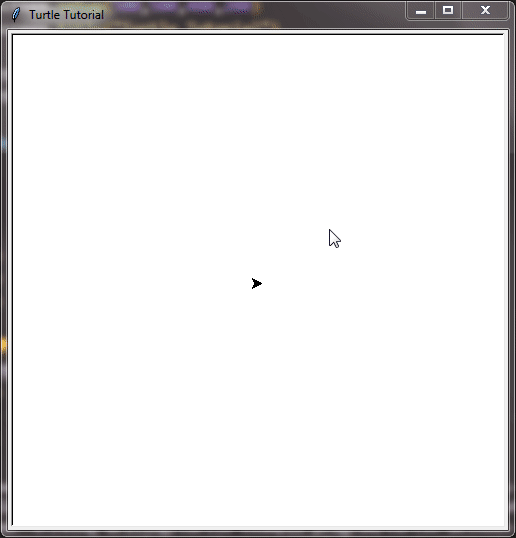
ondrag(func, btn, add)
= This function will bind the turtle with the mouse move event. Dragging the turtle with the mouse will invoke the func function.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() tt.speed(0) tt.ondrag(tt.goto) turtle.done()
Output :
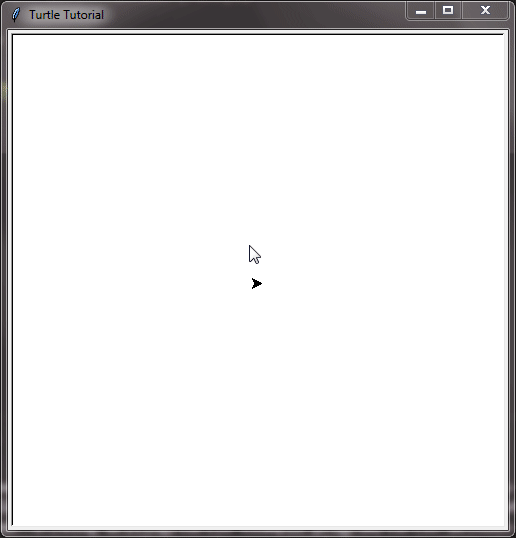
Functions For The Events On The Screen
onclick(func, btn, add)
= This function will call the func when you click the mouse button on the screen.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() tt.speed(0) screen.listen() screen.onclick(tt.goto) turtle.done()
Output :
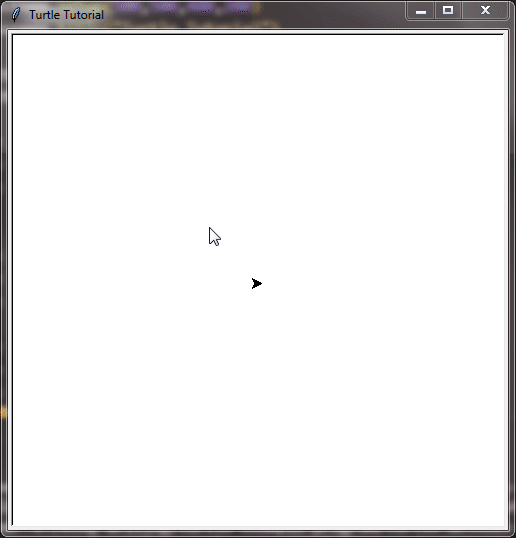
listen()
= This function will set focus on the screen for key events.
onkeypress(func, key)
= It will call the func when you press the specified key on the keyboard.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() tt.speed(0) def func(): tt.reset() tt.circle(100) screen.listen() screen.onkeypress(func,'c') turtle.done()
Output :
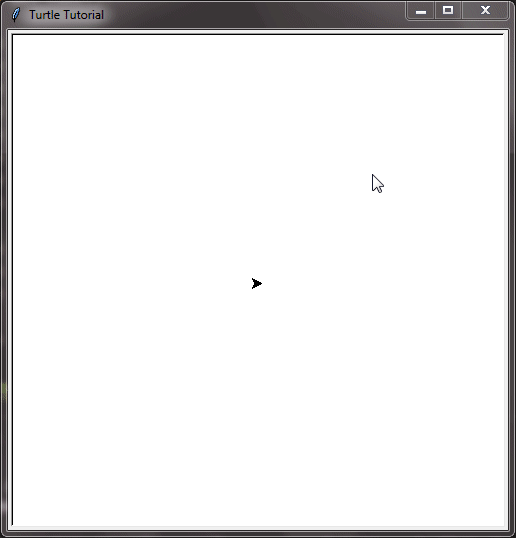
onkeyrelease(func, key)
= It will call the func when you release after pressing the specified key on the keyboard.
ontimer(func, t)
= This function will call func after every given t milliseconds.
Example :
import turtle screen=turtle.Screen() screen.setup(500,500,400,200) screen.title("Turtle Tutorial") tt=turtle.Turtle() screen.listen() tt.speed("fast") tt.penup() def func(): if tt.pos()[0] == 200 and tt.pos()[1] == 0: tt.setheading(90) if tt.pos()[0] == 200 and tt.pos()[1] == 200: tt.setheading(180) if tt.pos()[0] == 0 and tt.pos()[1] == 200: tt.setheading(270) if tt.pos()[0] == 0 and tt.pos()[1] == 0: tt.clear() tt.forward(2) screen.ontimer(func,1) screen.onkeyrelease(func,'s') turtle.done()
Output :
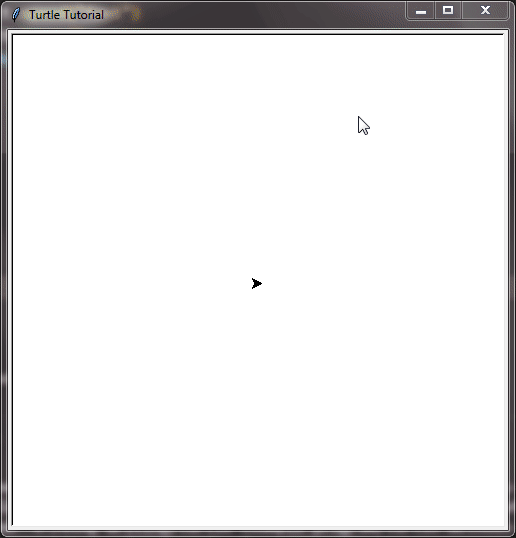