Python os.path Module Tutorial
The os.path
module contains functions for working with file paths and directories. It’s designed to handle file path operations in a way that’s compatible with different operating systems, such as Windows, Linux, and macOS. This means you can write code that deals with file paths in a consistent way, regardless of the underlying operating system.
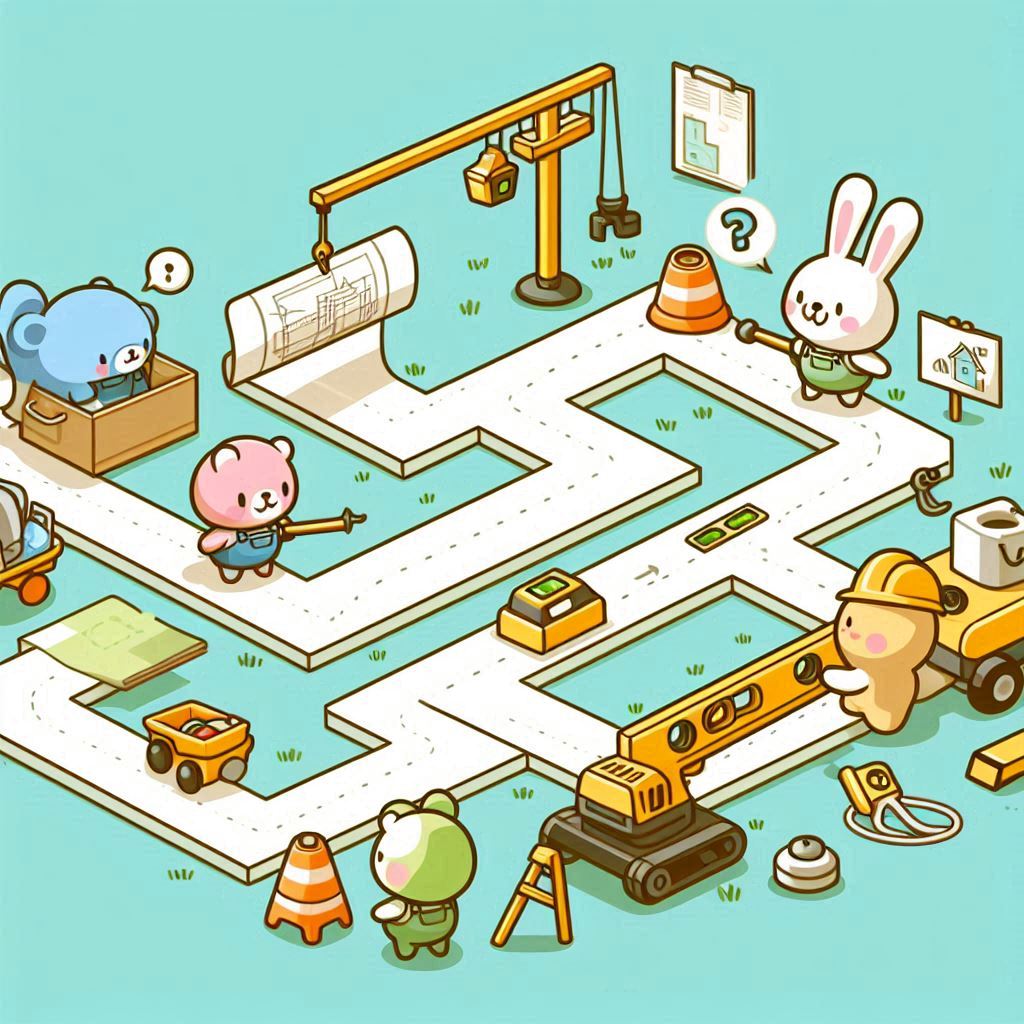
Finding Absolute Paths with os.path.abspath()
An absolute path refers to the complete path of a file or directory, starting from the root of the file system. The os.path.abspath()
function takes a relative or partial path as input and returns its absolute equivalent.
Absolute paths are commonly used in configuration files to specify the locations of files or directories.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_2=os.path.abspath('file2.txt') absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(absolute_path_1,absolute_path_2,absolute_path_3,absolute_path_4,absolute_path_5,sep="\n")
Output:
E:\blog writing\os example program\ospathfolder\file.txt
E:\blog writing\os example program\ospathfolder\file2.txt
E:\blog writing\os example program\ospathfolder\file3.txt
E:\blog writing\os example program\ospathfolder\sampledir
E:\blog writing\os example program\ospathfolder\sampledir\file4.txt
Extracting Directory Names
The os.path.basename()
function extracts the last component of a file path, which typically represents the name of a file or directory. In simpler terms, it gives you the name of the file or the last folder in a path.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_4=os.path.abspath('sampledir') print(os.path.basename(absolute_path_1)) print(os.path.basename(absolute_path_4))
Output:
file.txt
sampledir
On the other hand, the os.path.dirname()
function extracts the directory component of a file path, which represents the path to the parent directory of the specified file or directory.
Example:
import os absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.dirname(absolute_path_3)) print(os.path.dirname(absolute_path_4)) print(os.path.dirname(absolute_path_5))
Output:
E:\blog writing\os example program\ospathfolder
E:\blog writing\os example program\ospathfolder
E:\blog writing\os example program\ospathfolder\sampledir
Discovering Common Paths
The os.path.commonpath()
function is useful for identifying the common base path shared among multiple paths. It operates by taking a list of paths as input and determining the longest common directory path that all provided paths share.
In contrast, the os.path.commonprefix()
method focuses on the initial characters of each path and identifies the longest portion that remains consistent across all paths. It doesn’t differentiate between directories and files; instead, it solely examines the characters at the beginning of each path.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.commonpath((absolute_path_1,absolute_path_4,absolute_path_5))) print(os.path.commonprefix((absolute_path_1,absolute_path_4,absolute_path_5)))
Output:
E:\blog writing\os example program\ospathfolder
E:\blog writing\os example program\ospathfolder\
Know the Size, and Checking Access and Modification Times
The os.path.getsize()
helps you to measure how much space a file takes up on your computer. It simply tells you the size of a file in bytes.
If you’re curious about when a file was last opened or changed, os.path.getatime()
and os.path.getmtime()
functions can help you with that. They return timestamps indicating when the file was last accessed or modified.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_2=os.path.abspath('file2.txt') absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') print(os.path.getatime(absolute_path_4)) print(os.path.getctime(absolute_path_3)) print(os.path.getmtime(absolute_path_2)) print(os.path.getsize(absolute_path_1))
Output:
1669043896.072753
1669043896.072753
1668889326.1595917
112
Knowing the Nature of Paths
The os.path.isabs(path)
function checks if a path is absolute, meaning it starts from the root of the file system. If the path is absolute, it returns True
; otherwise, it returns False
.
Use the os.path.isdir(path)
function to check if a path refers to a directory. If the path points to a directory, it returns True
; otherwise, it returns False
. Similarly, the os.path.isfile(path)
function determines if a path points to a regular file.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_2=os.path.abspath('file2.txt') absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print("Result of isabs() :") print(os.path.isabs(os.path.basename(absolute_path_1))) print(os.path.isabs(absolute_path_2)) print("Result of isdir() :") print(os.path.isdir(absolute_path_4)) print(os.path.isdir(absolute_path_3)) print("Result of isfile() :") print(os.path.isfile(absolute_path_5)) print(os.path.isfile(absolute_path_4))
Output:
Result of isabs() :
False
True
Result of isdir() :
True
False
Result of isfile() :
True
False
Joining and Splitting Paths
Sometimes you need to combine multiple path components into one or break a path into its parts. That’s where these functions come in:
You can use the os.path.join()
function to combine multiple path components into one. It handles the differences in path separators between operating systems for you.
Example:
import os absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.join(absolute_path_4,absolute_path_3)) print(os.path.join(absolute_path_5,'sample')) print(os.path.join(absolute_path_4,'file4.txt'))
Output:
E:\blog writing\os example program\ospathfolder\file3.txt
E:\blog writing\os example program\ospathfolder\sampledir\file4.txt\sample
E:\blog writing\os example program\ospathfolder\sampledir\file4.txt
If you need to break a path into its directory and file parts, the os.path.split()
function does just that. It splits a path into two parts and gives you the directory part and the file part separately.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.split(absolute_path_1)) print(os.path.split(absolute_path_5))
Output:
('E:\\blog writing\\os example program\\ospathfolder', 'file.txt')
('E:\\blog writing\\os example program\\ospathfolder\\sampledir', 'file4.txt')
When you want to separate the base name of a file from its extension, the os.path.splitext()
function comes in handy. It splits a path into its base name and extension.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.splitext(absolute_path_1)) print(os.path.splitext(absolute_path_5))
Output:
('E:\\blog writing\\os example program\\ospathfolder\\file', '.txt')
('E:\\blog writing\\os example program\\ospathfolder\\sampledir\\file4', '.txt')
If your path includes a drive (like "C:\"
on Windows), the os.path.splitdrive()
function helps you separate the drive part from the rest of the path and returns both as a tuple.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.splitdrive(absolute_path_1)) print(os.path.splitdrive(absolute_path_5))
Output:
('E:', '\\blog writing\\os example program\\ospathfolder\\file.txt')
('E:', '\\blog writing\\os example program\\ospathfolder\\sampledir\\file4.txt')
Path Existence and Normalization
Before performing operations on files or directories, it’s crucial to check if the paths exist. The os.path.exists()
function allows you to do just that. If the path exists, it returns True
; otherwise, it returns False
.
Example:
import os absolute_path_3=os.path.abspath('file3.txt') absolute_path_4=os.path.abspath('sampledir') absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(absolute_path_3) print(os.path.exists(absolute_path_3)) print(absolute_path_5) print(os.path.exists(absolute_path_5)) print(os.path.join(absolute_path_4,'sample')) print(os.path.exists(os.path.join(absolute_path_4,'sample')))
Output:
E:\blog writing\os example program\ospathfolder\file3.txt
True
E:\blog writing\os example program\ospathfolder\sampledir\file4.txt
True
E:\blog writing\os example program\ospathfolder\sampledir\sample
False
Path normalization means making paths consistent across different platforms so that they’re formatted correctly and can be easily manipulated. Here are the functions that help with this:
Use os.path.normpath(path)
function to clean up a path by removing any unnecessary parts and ensuring it’s correctly formatted.
On Windows systems, where file paths aren’t case-sensitive, the os.path.normcase(path)
function makes all path components have consistent casing. It also converts forward slashes into backslashes.
Example :
import os absolute_path_2=os.path.abspath('file2.txt') print(os.path.normpath(absolute_path_2)) print(os.path.normcase(absolute_path_2))
Output:
E:\blog writing\os example program\ospathfolder\file2.txt
e:\blog writing\os example program\ospathfolder\file2.txt
Similar to os.path.abspath()
, the os.path.realpath(path)
function returns the absolute path, resolving any symbolic links.
When you need to find the relative path between two locations, the os.path.relpath(path)
function computes it for you.
Example:
import os absolute_path_5=os.path.abspath('sampledir\\file4.txt') print(os.path.relpath(absolute_path_5)) print(os.path.realpath(os.path.relpath(absolute_path_5)))
Output:
sampledir\file4.txt
E:\blog writing\os example program\ospathfolder\sampledir\file4.txt
Comparing Paths
The os.path.samefile(path1, path2)
function checks if two paths point to the same file or directory. If they do, it returns True
; otherwise, it returns False
.
Example:
import os absolute_path_1=os.path.abspath('file.txt') absolute_path_2=os.path.abspath('file2.txt') print(os.path.samefile(absolute_path_1,absolute_path_2)) print(os.path.samefile(absolute_path_1,"file.txt"))
Output:
False
True