Decision-Making Statements in Python
Decision-making statements let your program decide what to do based on certain conditions. They control the flow of your code, making it more dynamic and responsive. Let’s break down the different types of decision-making statements in Python.
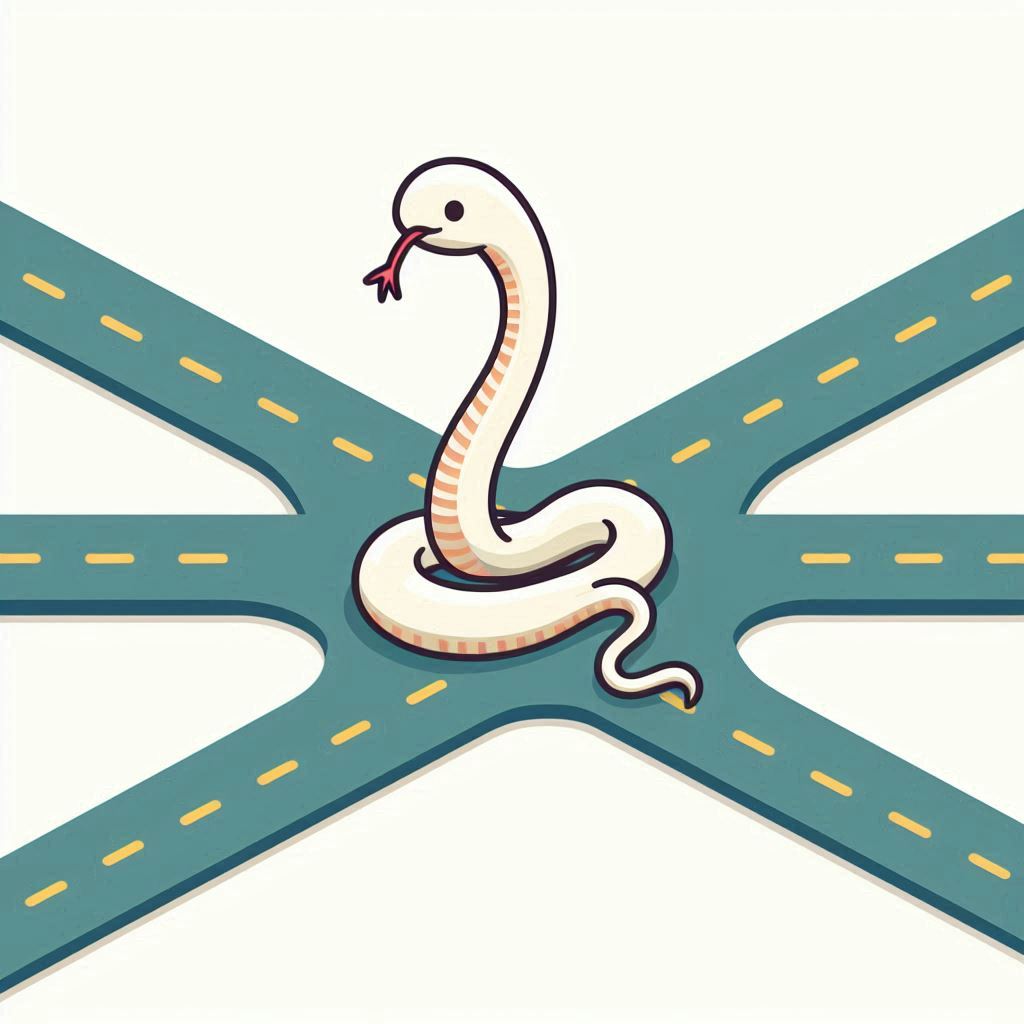
Table of Contents
The if Statement
The if
statement checks a condition. If the condition is True
, it runs a block of code. If the condition is False
, it skips the block.
Syntax:
if condition: # code block to run if the condition is True
Example:
# if statement x = 10 if x > 5: print("x is greater than 5") # Executed if the condition is true
Output:
x is greater than 5
if-else Statement
The if-else
statement provides an alternative block of code if the condition is False
.
Syntax:
if condition: # code block to run if the condition is True else: # code block to run if the condition is False
Example:
x = 3 if x > 5: print("x is greater than 5") else: print("x is not greater than 5")
Output:
x is not greater than 5
if-elif-else Statement
The if-elif-else
statement lets you check multiple conditions. The first condition that is True
will have its block of code executed. If none of the conditions are True
, the else
block runs.
Syntax:
if condition1: # code block to run if condition1 is True elif condition2: # code block to run if condition2 is True else: # code block to run if none of the conditions are True
Example:
x = 7 if x > 10: print("x is greater than 10") elif x > 5: print("x is greater than 5 but less than or equal to 10") else: print("x is 5 or less")
Output:
x is less than 10
Using Comparison Operators with if Statements
Comparison operators are used to compare two values or expressions. They are often used in if
statements to form conditions.
Example:
x = 10 y = 5 if x == y: print("x is equal to y") elif x != y: print("x is not equal to y") if x > y: print("x is greater than y") if x < y: print("x is less than y") if x >= y: print("x is greater than or equal to y") if x <= y: print("x is less than or equal to y")
Output:
x is not equal to y
x is greater than y
x is greater than or equal to y
Using Logical Operators in Conditions
Logical operators combine multiple conditions in decision-making statements. The most common logical operators are and
, or
, and not
.
Example:
x = 10 y = 5 z = 15 # Using 'and' operator if x > y and x < z: print("x is greater than y and less than z") # Using 'or' operator if x > y or x > z: print("x is greater than y or z") # Using 'not' operator if not x == y: print("x is not equal to y")
Output:
x is greater than y and less than z
x is greater than y or z
x is not equal to y
Nested if Statements
A nested if
statement is an if
statement inside another if
statement. This allows you to check multiple conditions sequentially.
Syntax:
if condition1: # code block for condition1 if condition2: # code block for condition2
Example:
x = 10 y = 5 if x > 5: print("x is greater than 5") if y < 10: print("y is less than 10")
Output:
x is greater than 5
y is less than 10
Conditional Expression
A conditional expression in Python allows you to write a compact if-else
statement in a single line. It is often used for simple conditions to make the code more concise.
Syntax:
value_if_true if condition else value_if_false
Example:
x = 10 # Regular if-else statement if x > 5: result = "Greater" else: result = "Lesser" # Conditional expression result = "Greater" if x > 5 else "Lesser" print(result)
Output:
Greater
Introduction to match-case Statement
Python 3.10 introduced a new way to handle multiple conditions called the match-case
statement. It’s similar to switch-case statements in other programming languages and helps make your code more organized and easier to read.
Syntax:
The match-case
statement uses pattern matching to decide which block of code to execute. Here’s how it looks:
match variable: case pattern1: # Code block for pattern1 case pattern2: # Code block for pattern2 case _: # Code block if no other pattern matches (default case)
Example:
status_code = 404 match status_code: case 200: result = "OK" case 404: result = "Not Found" case 500: result = "Server Error" case _: result = "Unknown Status" print(result)
Output:
Not Found
Use Cases of match-case
- Easily map different HTTP response codes to messages or actions.
- Simplify user input handling in menu-driven programs.
- Make state transitions in your code more readable.
Lambda Functions with Decision-Making
Lambda functions are small, anonymous functions created with the lambda
keyword. They’re handy for writing concise, one-liner functions, especially when combined with conditional expressions.
Example:
x = 10 # Regular if-else statement def check_value(x): return "Greater" if x > 5 else "Lesser" print(check_value(x)) # Using a lambda function check_value_lambda = lambda x: "Greater" if x > 5 else "Lesser" print(check_value_lambda(x))
Output:
Greater
Greater
Handling Complex Decision Trees
Advanced nested conditions involve multiple layers of if-elif-else
statements to handle complex decision trees. These are often necessary when dealing with intricate logic that cannot be simplified with basic conditions or logical operators.
Example:
def assess_risk(age, health): if age < 30: if health == "good": return "Low Risk" elif health == "average": return "Moderate Risk" else: return "High Risk" elif 30 <= age < 60: if health == "good": return "Moderate Risk" else: return "High Risk" else: return "High Risk" print(assess_risk(25, "good")) print(assess_risk(45, "poor"))
Output:
Low Risk
High Risk
Best Practices
- Too many nested levels can make your code hard to read and maintain. Keep it simple.
- Break down complex logic into smaller functions to make your code modular and easier to understand.
- Explain the logic behind complex conditions with comments.