Formatting Strings: The Power of f-strings
String formatting in Python is a handy way to create and customize text, making it clear and easy to read. Whether you’re making user messages, logs, or reports, string formatting is a key skill for any Python programmer.
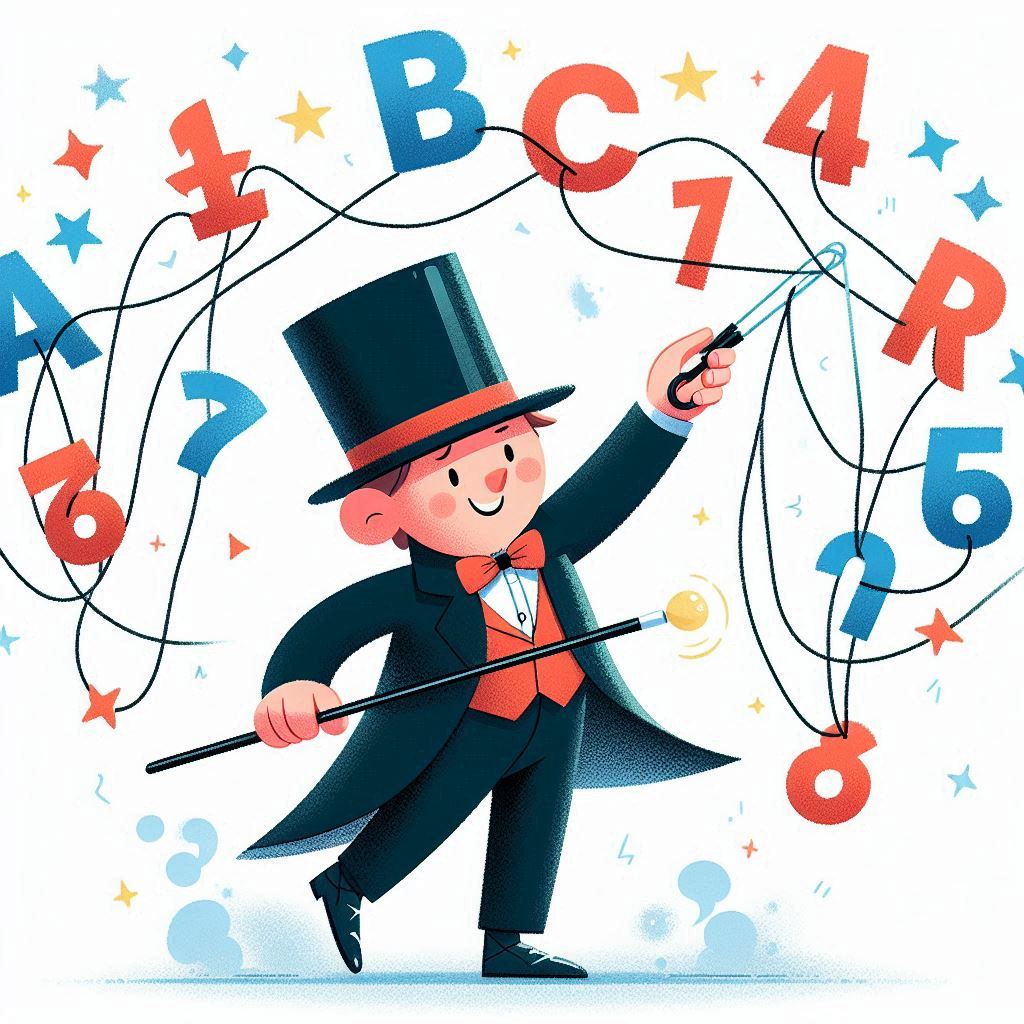
Basic String Formatting
There are a few basic ways to format strings in Python. Let’s look at some of them.
Concatenation Using +
Concatenation means joining strings together using the +
operator. This method is straightforward and works well for simple cases.
Example:
name = "Amit" message = "Hello, " + name print(message)
Output:
Hello, Amit
Using str() for Conversion
The str()
function is handy when you need to include non-string data (like numbers) in your strings. It converts different data types to strings.
Example:
price = 19.99 message = "The price of the item is " + str(price) + " dollars." print(message)
Output:
The price of the item is 19.99 dollars.
Old-style String Formatting (%)
Old-style string formatting, also known as printf-style formatting, uses the %
operator to insert values into a string. This method is similar to the way C handles string formatting and is still available in Python for backward compatibility.
Syntax
The general syntax for old-style string formatting involves placing format specifiers within the string, which are then replaced by corresponding values after the %
operator.
"string with %s specifier" % value
Format Specifiers
%s
– String (or any object with a string representation)%d
– Integer%f
– Floating-point number%x
– Hexadecimal integer
Let’s look at some examples demonstrating the use of old-style string formatting with different data types.
Example 1: Formatting Strings
The %s
format specifier is replaced by the value of name
.
name = "Amit" message = "Hello, %s!" % name print(message)
Output:
Hello, Amit!
Example 2: Formatting Integers
The %d
format specifier is replaced by the value of age
.
age = 25 message = "You are %d years old." % age print(message)
Output:
You are 25 years old.
Example 3: Formatting Floating-point Numbers
The %.2f
format specifier formats the float to 2 decimal places.
price = 19.99 message = "The price is %.2f dollars." % price print(message)
Output:
The price is 19.99 dollars.
Always, use the correct format specifier for the data type.
Example 4: Multiple Values
This example uses multiple format specifiers, and their corresponding values are provided as a tuple. Remember, the number of values matches the number of format specifiers otherwise, it will throw an error.
name = "Amit" age = 25 message = "Hello, %s! You are %d years old." % (name, age) print(message)
Output:
Hello, Amit! You are 25 years old.
New-style String Formatting (str.format())
The str.format()
method is a more powerful and flexible way to format strings in Python. It was introduced in Python 2.7 and 3.1 and offers several advantages over the older %
formatting.
Syntax
The basic syntax for str.format()
involves using curly braces {}
as placeholders within the string. These placeholders are replaced by the values passed to the format()
method.
"string with {} placeholder".format(value)
Example 1: Unnamed Placeholders
name = "Amit" age = 25 message = "Hello, {}! You are {} years old.".format(name, age) print(message)
Output:
Hello, Amit! You are 25 years old.
Example 2: Named Placeholders
message = "Hello, {name}! You are {age} years old.".format(name="Amit", age=25) print(message)
Output:
Hello, Amit! You are 25 years old.
Example 3: Nested and Complex Formatting
New-style formatting supports nested and complex expressions within the placeholders.
data = {"name": "Amit", "age": 25} message = "Hello, {name}! You are {age} years old.".format(**data) print(message)
Output:
Hello, Amit! You are 25 years old.
Example 4: Alignment, Padding, and Width
You can control the alignment, padding, and width of the formatted output using format specifiers inside the curly braces.
- Alignment:
<
(left),>
(right),^
(center) - Padding: Use any character to pad the value.
- Width: Specify the minimum width of the output.
message = "{:<10} | {:^10} | {:>10}".format("left", "center", "right") print(message)
Output:
left | center | right
Example 5: Formatting Numbers, Dates, and Other Data Types
You can format numbers, dates, and other data types using format specifiers.
from datetime import datetime now = datetime.now() formatted_date = "{:%Y-%m-%d %H:%M}".format(now) print(formatted_date)
Output:
2024-07-02 23:15
Example 6: Using Nested Fields
data = {"user": {"name": "Amit", "balance": 1234.56}} message = "Hello, {user[name]}! Your balance is {user[balance]:,.2f}.".format(**data) print(message)
Output:
Hello, Amit! Your balance is 1,234.56.
Introduction to f-strings
f-strings, or formatted string literals, were introduced in Python 3.6 to provide a more readable and concise way to format strings. They allow embedding expressions inside string literals, using curly braces {}
.
Syntax
To create an f-string, prefix the string with the letter f
or F
. Inside the string, you can place any valid Python expression within curly braces {}
, and the expression will be evaluated and formatted.
Example:
name = "Amit" age = 25 message = f"Hello, {name}! You are {age} years old." print(message)
Output:
Hello, Amit! You are 25 years old.
f-strings are generally faster because they are evaluated at runtime.
Embedding Expressions in f-strings
You can embed various types of expressions inside f-strings, including arithmetic operations, function calls, and more.
Example 1: Arithmetic Expressions
a = 5 b = 10 result = f"The sum of {a} and {b} is {a + b}." print(result)
Output:
The sum of 5 and 10 is 15.
Example 2: Function Calls
import math radius = 3 area = f"The area of a circle with radius {radius} is {math.pi * radius ** 2:.2f}." print(area)
Output:
The area of a circle with radius 3 is 28.27.
Example 3: Access variables and attributes
F-strings also allow you to access variables and attributes within complex objects effortlessly.
Example:
# Accessing variables and attributes person = { "name": "Bob", "age": 30, "address": { "street": "123 Main St", "city": "New York" } } message = f"Hello, {person['name']}! You live in {person['address']['city']}." print(message) # Output: "Hello, Bob! You live in New York."
Output:
Hello, Bob! You live in New York.
Formatting with f-strings
You can control the formatting of numbers, dates, and strings using format specifiers inside f-strings. This includes specifying the width, precision, and type of the formatted output.
Example 1: Formatting Numbers
num = 12345 float = f"float representation: {number:.3f}" decimal = f"Decimal representation: {num:d}" hexadecimal = f"Hexadecimal representation: {num:x}" scientific = f"Scientific notation: {num:e}" print(float) print(decimal) print(hexadecimal) print(scientific)
Output:
float representation: 1234.568
Decimal representation: 12345
Hexadecimal representation: 3039
Scientific notation: 1.234500e+04
Example 2: Formatting Dates
from datetime import datetime now = datetime.now() formatted_date = f"{now:%Y-%m-%d %H:%M:%S}" print(formatted_date)
Output:
2024-07-02 23:42:32
Example 3: Alignment and Padding with f-strings
Like str.format()
, you can align and pad your strings using format specifiers inside f-strings.
name = "Amit" left_aligned = f"{name:<10}" right_aligned = f"{name:>10}" center_aligned = f"{name:^10}" print(left_aligned) print(right_aligned) print(center_aligned)
Output:
Amit
Amit
Amit