Lists And Tuples in Python
Python offers two primary data structures for storing collections of items: lists and tuples. Both allow you to store multiple items in a single variable, but they have different properties and use cases.
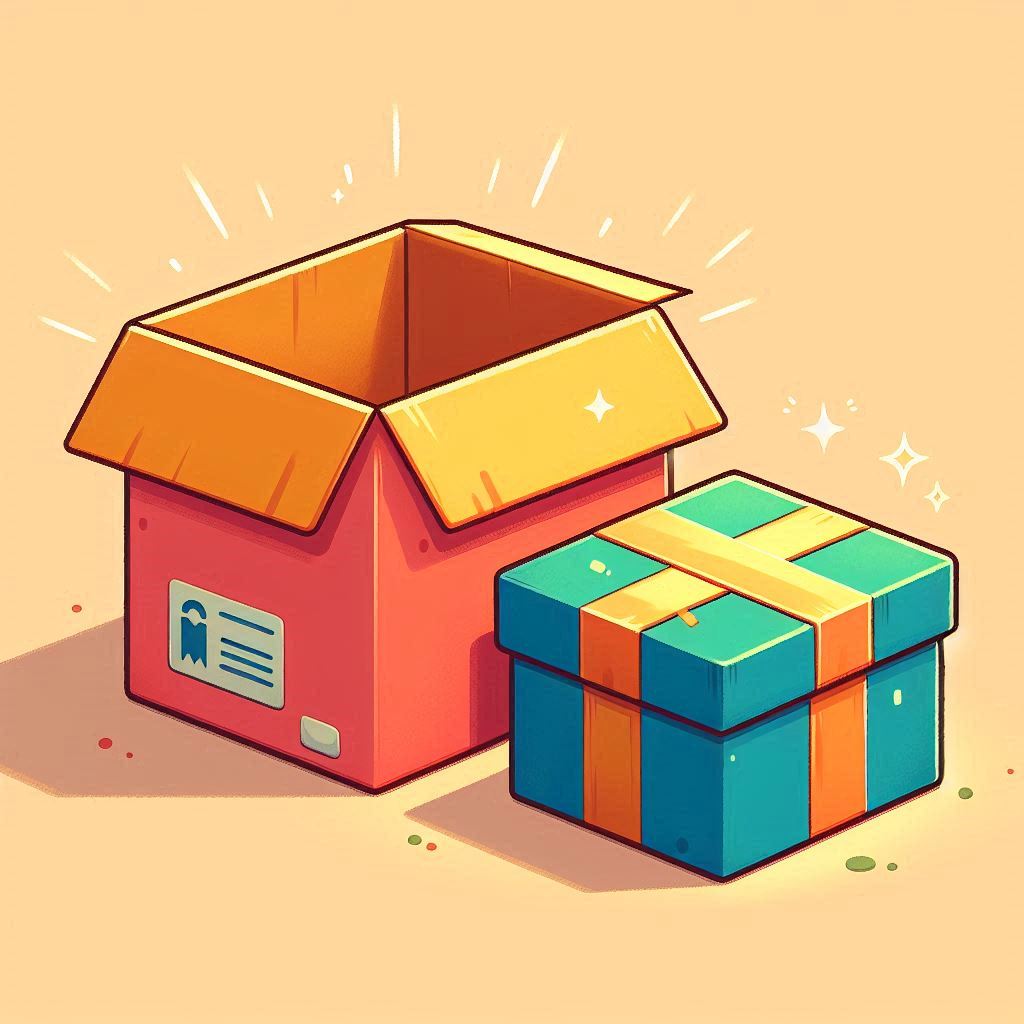
A list in Python is a collection of items that are ordered and can be changed. You can store different types of data in a single list, such as numbers, strings, or even other lists. Lists are great for tasks where you need to add, remove, or modify items frequently
Tuples are like lists but are immutable which means you cannot change them after you create them. It would be best to use a tuple when you have a fixed set of items. For example, storing days of the week or a collection of constants that shouldn’t change.
Understanding these structures will help you write better Python programs.
Creating Lists in Python
To create a list, we have to enclose items within square brackets []
and each item is separated by a comma.
Example:
# Creating lists numbers = [1, 2, 3, 4, 5] fruits = ["apple", "banana", "orange"] mixed = [10, "hello", True, 3.14] print(numbers) print(fruits) print(mixed)
In this example, we’ve created three lists: numbers
, fruits
, and mixed
. The numbers
list holds integers, the fruits
list is all about strings, and the mixed
list contains different data types!
Output:
[1, 2, 3, 4, 5]
['apple', 'banana', 'orange']
[10, 'hello', True, 3.14]
You can also create an empty list and add items later.
Example:
# Creating an empty list empty_list = []
Inside a list, you can contain other lists, which is useful for representing matrices or tables. Here’s how:
Example:
# Creating a nested list matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] print(matrix)
Output:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
Access List Elements
Lists are ordered collections, which means each element has a specific position, starting from 0. You can access elements by their index.
Example:
# Accessing list elements numbers = [1, 2, 3, 4, 5] fruits = ["apple", "banana", "orange"] first_number = numbers[0] # Accessing the first element: 1 second_fruit = fruits[1] # Accessing the second element: "banana" print(first_number) print(second_fruit)
Output:
1
banana
Negative Indexing
You can also access elements by negative indexing, where -1
refers to the last element, -2
to the second last, and so on.
Example:
numbers = [1, 2, 3, 4, 5] fruits = ["apple", "banana", "orange"] second_last_number = numbers[-2] last_fruit = fruits[-1] print(second_last_number) print(last_fruit)
Output:
4
orange
Slicing a List
By using the slicing techniques, you can access a range of elements.
The syntax for slicing is list[start:stop:step]
.
start
: This is the index where the slice starts (inclusive). If omitted, the slice starts from the beginning of the list.
stop
: This is the index where the slice ends (exclusive). If omitted, the slice goes to the end of the list.
step
: This specifies the interval between elements. If you don’t provide this, the default value is 1, which means it includes every element between start
and stop
.
Example:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Slice from start to stop slice1 = numbers[2:5] print(slice1) # Slice from start to end slice2 = numbers[3:] print(slice2) # Slice from beginning to stop slice3 = numbers[:4] print(slice3) # Slice with a positive step slice4 = numbers[1:7:2] print(slice4) # Slice with a negative step slice5 = numbers[7:1:-2] print(slice5) # Omitting start and stop slice6 = numbers[::2] print(slice6) # Reversing the list slice7 = numbers[::-1] print(slice7)
Output:
[3, 4, 5]
[4, 5, 6, 7, 8, 9, 10]
[1, 2, 3, 4]
[2, 4, 6]
[8, 6, 4]
[1, 3, 5, 7, 9]
[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]
Modifying List Elements
Lists in Python are mutable, which means their elements can be changed. You can change the item at a specific index by using its index value.
Example:
# Modifying list elements fruits = ["apple", "banana", "orange"] fruits[1] = "grape" # Modifying the second element: ["apple", "grape", "orange"] print(fruits)
Output:
['apple', 'grape', 'orange']
List Operations
Python lists support several operations that make it easy to manipulate and work with lists.
Concatenation: You can concatenate two or more lists using the +
operator. This operation combines two or more lists into a single big list.
Repetition: You can repeat the list items inside the list, multiple times by using the *
operator.
Length: By using the len()
function, you can find how many items, your list contains.
Example:
# List operations list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list1 + list2 repeated_list = list1 * 3 length_of_list = len(list1) print(concatenated_list) print(repeated_list) print(length_of_list)
Output:
[1, 2, 3, 4, 5, 6]
[1, 2, 3, 1, 2, 3, 1, 2, 3]
3
Add Items to the List
If you want to add a new item to your list, Python provides several methods to do this:
append()
: You can add a new item to the end of the list.
insert()
: With this, you can insert a new item at a specific position in the list.
extend()
: It helps you to add multiple items to the end of the list.
Example:
fruits = ['apple', 'banana', 'cherry'] # Let's add some fruits to our list fruits.append("orange") print(fruits) fruits.insert(1, "grapes") print(fruits) fruits.extend(["watermelon", "mango"]) print(fruits)
Output:
['apple', 'banana', 'cherry', 'orange']
Remove Items from the List
If you want to get rid of items from your list, you have a few ways to do it:
remove()
: This method allows you to specify the item you want to remove from the list.
pop()
: With this method, you can specify the position of the item you want to remove, and it will return that item. If you don’t give any index, it will remove the last item.
del
: This keyword allows you to delete an item at a specific position or a range of items from the list.
clear()
: This method removes all items from the list, leaving it empty.
Example:
fruits = ['apple', 'grapes', 'banana', 'cherry', 'orange', 'watermelon', 'mango'] # Let's say we don't want "watermelon" anymore fruits.remove("watermelon") print(fruits) # Let's remove "grapes" from the second position removed_fruit = fruits.pop(1) print(fruits) print(removed_fruit) # Let's delete "apple" from the first position and "orange" from the fourth position del fruits[0] del fruits[2] print(fruits) # Let's clear the entire list fruits.clear() print(fruits)
Output:
['apple', 'grapes', 'banana', 'cherry', 'orange', 'mango']
['apple', 'banana', 'cherry', 'orange', 'mango']
grapes
['banana', 'cherry', 'mango']
[]
Other Useful Methods for Lists
Apart from the basic methods we’ve already discussed for managing lists, Python offers several other helpful methods to make your list manipulation tasks even easier.
Finding Elements with index()
If you want to know the position of a specific item in your list, the index()
method helps you with that. It tells you the position of the first occurrence of an item.
Example:
fruits = ['apple', 'banana', 'cherry'] index = fruits.index('banana') print(index)
Output:
1
Counting Occurrences with count()
How many times does a particular item show up in your list? The count()
method gives you the answer by counting the occurrences.
Example:
numbers = [1, 2, 2, 3, 2, 4, 2] count = numbers.count(2) print(count)
Output:
4
Sorting Your List with sort()
Sorting a list alphabetically or numerically is a common task. The sort()
method does just that, arranging your items in ascending order.
Example:
# Let's sort a list of numbers numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3] numbers.sort() print(numbers) # We can also sort in descending order numbers.sort(reverse=True) print(numbers) # Sorting strings alphabetically fruits = ["banana", "apple", "cherry"] fruits.sort() print(fruits)
Output:
[1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
[9, 6, 5, 5, 4, 3, 3, 2, 1, 1]
['apple', 'banana', 'cherry']
Finding Elements with index()
The reverse()
method reverses the order of the elements in the list.
Example:
fruits = ['apple', 'banana', 'cherry'] fruits.reverse() print(fruits)
Output:
['cherry', 'banana', 'apple']
Making a Copy with copy()
When you need to work with a list without modifying the original, the copy()
method comes in handy. The copy()
method helps you create a duplicate list without affecting the original.
Example:
numbers = [1, 2, 3] numbers_copy = numbers.copy() print(numbers_copy)
Output:
[1, 2, 3]
Creating Tuples in Python
In Python, you can create a tuple by placing values separated by commas inside parentheses ()
.
Example:
In the example, we’re creating three tuples: point
, colors
, and mixed
.
# Creating tuples point = (3, 4) colors = ("red", "green", "blue") mixed = (10, "hello", True) print(point) print(colors) print(mixed)
Output:
(3, 4)
('red', 'green', 'blue')
(10, 'hello', True)
If you want to create a tuple with just one element, then you can create it by adding a comma after the element. Without the comma, Python will not recognize it as a tuple.
Example:
# Single-element tuple single_element_tuple = (1,) print(single_element_tuple) # Output: (1,)
Tuple Packing and Unpacking
Tuple Packing means we can create a tuple without using parentheses ()
.
Unpacking is taking values out of a tuple and assigning them to variables. The number of variables must match the number of items in the tuple.
Example:
# Tuple packing and unpacking x = 10 y = 20 z = 30 coordinates = x, y, z # Tuple packing: (10, 20, 30) a, b, c = coordinates # Tuple unpacking: a = 10, b = 20, c = 30 print(a, b, c)
Output:
10 20 30
You can also use the *
operator to unpack the remaining elements into a list.
Example:
# Unpacking with the * operator fruits = ("apple", "banana", "watermelon", "grapes") fruit1, *remaining_fruits = fruits print(fruit1) print(remaining_fruits)
Output:
apple
['banana', 'watermelon', 'grapes']
Access Tuple Items
You can access elements in a tuple just like you do with lists, using indexes. Indexes start at 0, so the first element is at index 0.
Example:
# Accessing tuple elements point = (3, 4) colors = ("red", "green", "blue") x = point[0] # Accessing the first element: 3 second_color = colors[1] # Accessing the second element: "green" print(x) print(second_color)
Output:
3
green
Use Negative Index
Negative indexes let you access elements from the end of the tuple.
Example:
fruits = ("apple", "banana", "cherry") print(fruits[-1]) print(fruits[-2]) print(fruits[-3])
Output:
cherry
banana
apple
Slice a Tuple
You can slice a tuple too to get a range of items. The syntax is the same as of the list – tuple[start:stop:step]
.
Example:
fruits = ("apple", "banana", "orange", "pineapple", "watermelon", "grape") # Slicing from index 1 to 4 print(fruits[1:4]) # Slicing with a step print(fruits[0:6:2])
Output:
('banana', 'orange', 'pineapple')
('apple', 'orange', 'watermelon')
Tuple Operations
Although tuples are immutable, meaning you cannot change their elements after they are created, you can still perform various operations such as concatenation (+
), repetition (*
), and finding their length (len()
).
Example:
In the example, we’re joining tuples, making copies of items, and counting the items.
# Tuple operations tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) concatenated_tuple = tuple1 + tuple2 # Concatenating two tuples: (1, 2, 3, 4, 5, 6) repeated_tuple = tuple1 * 3 # Repeating a tuple: (1, 2, 3, 1, 2, 3, 1, 2, 3) length_of_tuple = len(tuple1) # Determining the length of a tuple: 3 print(concatenated_tuple) print(repeated_tuple) print(length_of_tuple)
Output:
(1, 2, 3, 4, 5, 6)
(1, 2, 3, 1, 2, 3, 1, 2, 3)
3
Tuple Methods
Tuples indeed have fewer methods compared to lists, but the ones they do have are quite handy.
The count()
method tells you how many times a particular value appears in a tuple. This can be really helpful when you need to count occurrences of a specific item.
The index()
method returns the first position of a specified value in the tuple. If the value isn’t found, it raises an error.
Example:
# Tuple methods numbers = (1, 2, 3, 4, 2, 5, 2) count_2 = numbers.count(2) # Counting occurrences of 2: 3 index_4 = numbers.index(4) # Finding the index of 4: 3 print(count_2) print(index_4)
Output:
3
3