Python Built-in Functions
Python comes with many built-in functions. These functions are ready to use, which means you don’t need to import any modules or libraries to use them. They make coding easier and more efficient by providing simple ways to perform common tasks.
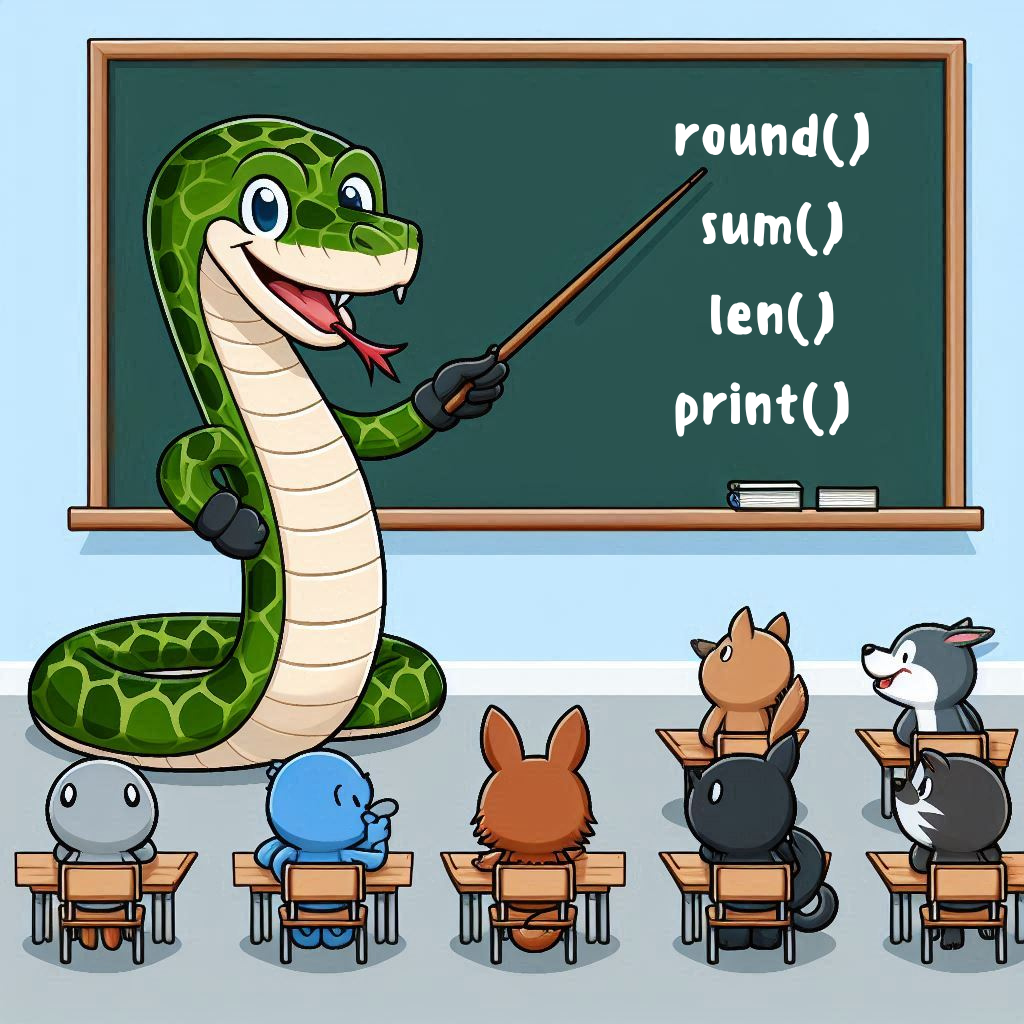
These built-in functions can be grouped into several categories based on what they do.
Here are a few major categories:
Input/Output Functions
The input/output (I/O) functions are like the communication channels between your program and the user. They allow your program to display information and receive input from the user.
Two key functions for this are print()
and input()
.
The print() Function
The print()
function is used to display output to the console or write to a file. It allows you to print strings, numbers, variables, and more.
Here’s the syntax:
print(*objects, sep=' ', end='\n', file=sys.stdout)
objects
: These are the values to be printed. You can print multiple objects by separating them with commas.
sep
: This parameter specifies the separator between the objects if there are multiple objects to be printed. By default, it’s a space (' '
).
end
: It specifies what to print at the end. The default is a newline character ('\n'
), which means the next print()
statement will start from a new line.
file
: You can specify a file object(like a text file) to which the output should be written. By default, the output goes to the console (sys.stdout
).
Example 1: Printing a simple string
print("Hello, World!")
Output:
Hello, World!
Example 2: Printing multiple objects with a custom separator
name = "John" age = 25 print("Name:", name, "Age:", age, sep=", ")
Output:
Name:, John, Age:, 25
Example 3: Writing output to a file
with open("output.txt", "w") as file: print("This is written to a file.", file=file) # Contents of output.txt: This is written to a file.
The input() Function
When you want your program to ask the user for something, you use the input()
function. It waits for the user to type something and then captures it as a string. You can pass only one parameter to this function.
– prompt
: Optional. This is an optional message that appears to the user, asking them for input like you can ask the user his name. Here’s a simple example:
Example:
name = input("Enter your name: ") print("Hello, " + name + "!")
Output:
Enter your name: Richard
Hello, Richard!
The input()
function always returns a string, but if you want to change the data type of user input, you can do this:
Example:
age = int(input("Enter your age: ")) print("Next year, you will be", age + 1, "years old.")
Output:
Enter your age: 23
Next year, you will be 24 years old.
Numeric Functions
Python has several built-in functions that help you perform common mathematical operations easily.
abs()
The abs()
function returns the absolute value of a number. The absolute value is the distance of a number from zero, ignoring whether it’s positive or negative.
Example:
x = -10 abs_x = abs(x) print(abs_x)
Output:
10
pow()
The pow()
function raises one number (the base) to the power of another number (the exponent). It’s a way to do exponentiation. It takes three parameters:
base
: The number you want to raise.
exp
: The power to which you want to raise the base.
mod
(optional): A number to divide the result by and return the remainder (useful in certain mathematical calculations).
Example:
base = 2 exponent = 3 result = pow(base, exponent) print(result) # With modulo result_modulo = pow(base, exponent, 5) print(result_modulo) # Output: 3 (8 % 5 = 3)
Output:
8
3
round()
The round()
function rounds a floating-point number to a specified number of decimal places or the nearest integer if no decimal places are specified. It takes two parameters:
number
: The number you want to round.
ndigits
(optional) : The number of decimal places to round to. If omitted, the number is rounded to the nearest integer.
Example:
x = 3.14159 rounded = round(x, 2) print(rounded) # Without specifying ndigits rounded_whole = round(x) print(rounded_whole)
Output:
3.14
3
min()
The min()
function finds the smallest item in an iterable (like a list, or a tuple) or the smallest of multiple arguments. It takes four parameters:
iterable
: A collection of items to find the minimum from.
arg1, arg2, *args
: Two or more arguments to compare.
key
(optional) : A function that defines the sorting criteria.
default
(optional) : A default value to return if the iterable is empty.
Example:
numbers = [5, 2, 9, 1, 7] smallest = min(numbers) print(smallest) # Using multiple arguments a = 10 b = 3 c = 7 smallest_arg = min(a, b, c) print(smallest_arg)
Output:
1
3
max()
The max()
function finds the largest item in an iterable or the largest of multiple arguments. It will take the same parameters as the min()
function.
Example:
numbers = [5, 2, 9, 1, 7] largest = max(numbers) print(largest) # Using multiple arguments a = 10 b = 3 c = 7 largest_arg = max(a, b, c) print(largest_arg)
Output:
9
10
String Functions
Strings are sequences of characters and are a fundamental part of programming. Now, let’s explore the five important built in string functions: len()
, str()
, format()
, join()
, and split()
.
len()
The len()
function returns the length of a string, which is the number of characters in the string.
Example:
word = "Hello" length = len(word) print(length)
Output:
5
You can also find the length of a list using the len()
function.
Example:
numbers = [1, 2, 3, 4, 5] length = len(numbers) print(length)
Output:
5
str()
The str()
function converts a given value into a string. The value can be a number, a list, a boolean, etc. If no object is provided, it returns an empty string.
Example 1: Converting a number to a string
number = 8 string_number = str(number) print(string_number)
Output:
8
Example 2: Converting a list to a string
numbers = [1, 2, 3, 4, 5] string_numbers = str(numbers) print(string_numbers)
Output:
[1, 2, 3, 4, 5]
format()
The format()
function allows you to create formatted strings. It lets you place placeholders in a string, which are replaced by values provided in the format()
method.
Example:
name = "Ayesha" age = 20 message = "My name is {} and I'm {} years old.".format(name, age) print(message)
Output:
My name is Ayesha and I'm 20 years old.
join()
The join()
function concatenates (joins together) elements of an iterable (like a list or tuple) into a single string, with a specified separator between each element. It takes two parameters:
separator
: The string to place between each element in the resulting string.
iterable
: The iterable (like a list) whose elements you want to join.
Example 1: Joining strings in a list with a space separator
words = ["Hello", "world", "!"] sentence = " ".join(words) print(sentence)
Output:
Hello world !
Example 2: Joining characters in a string with a hyphen separator
word = "Python" hyphenated_word = "-".join(word) print(hyphenated_word)
Output:
P-y-t-h-o-n
split()
The split()
function divides a string into a list of substrings based on your chosen delimiter. It takes two parameters:
separator
(optional): The string to use as the delimiter. If not specified, any whitespace is used.
maxsplit
(optional): The maximum number of splits to do. The default is -1
, which means “all occurrences”.
Example 1: Splitting a string into words
sentence = "Hello world!" words = sentence.split() print(words)
Output:
['Hello', 'world!']
Example 2: Splitting a string using a comma as a delimiter
numbers = "1,2,3,4,5" split_numbers = numbers.split(",") print(split_numbers)
Output:
['1', '2', '3', '4', '5']
Other Built-in functions
Now, let’s explore some other useful built-in functions of Python.
range()
The range()
function creates a sequence of numbers. It’s often used in loops to repeat actions a certain number of times. Here’s the syntax:
range(start, stop, step)
start
(optional): The first number in the sequence. Default is 0.
stop
: The number to stop at (not included).
step
(optional): The difference between each number in the sequence. Default is 1.
Example:
for i in range(1, 6, 2): print(i)
In this example, the range(1, 6, 2)
generates the sequence [1, 3, 5]
. The loop iterates over these values and prints them.
Output:
1
3
5
sum()
The sum()
function adds up all the numbers in a list or other collection.
Example:
my_list = [1, 2, 3, 4, 5] total = sum(my_list) print(total)
Output:
15
reverse()
The reverse()
function helps you to flip the order of elements in a list.
Example:
my_list = [1, 2, 3, 4, 5] my_list.reverse() print(my_list)
In this example, the reverse()
function reverses the order of elements in the my_list
, resulting in [5, 4, 3, 2, 1].
Output:
[5, 4, 3, 2, 1]
enumerate()
The enumerate()
function adds a counter to a list or other collection and returns it as an enumerate object, so you know the position of each item.
Example:
my_list = ['apple', 'banana', 'orange'] for index, value in enumerate(my_list): print(index, value)
In this example, the enumerate()
function adds a counter to the my_list
. The loop iterates over the enumerated objects, printing the index and value of each element.
Output:
0 apple
1 banana
2 orange
sorted()
Lastly, we have sorted()
function. It sorts a list or other collection and returns a new sorted list. Here’s the syntax:
sorted(iterable, key, reverse)
iterable
: The collection to sort.
key
(optional): A function to customize the sorting. Default is None
.
reverse
(optional): If True
, sorts in descending order. Default is False
.
Example 1: Sorting a List
my_list = [5, 3, 1, 4, 2] sorted_list = sorted(my_list) print(sorted_list)
In this example, the sorted()
function sorts the my_list
in ascending order, and the sorted result is stored in the sorted_list
.
Output:
[1, 2, 3, 4, 5]
Example 2: Sorting with a Key Function
words = ["banana", "apple", "kiwi"] sorted_words = sorted(words, key=len) print(sorted_words)
In this example, we are sorting the list of words by their length.
Output:
['kiwi', 'apple', 'banana']
Example 3: Sorting in Reverse Order
numbers = [3, 1, 4, 1, 5, 9] sorted_numbers = sorted(numbers, reverse=True) print(sorted_numbers)
In this example, we are sorting the list of numbers in descending order.
Output:
[9, 5, 4, 3, 1, 1]