Mastering Python Functions: Syntax, Parameters, Return Values, Lambda, Scope and more
A function is a block of code that performs a specific task. You can reuse this block of code whenever you need it, which saves time and makes your code cleaner. Instead of writing the same code multiple times, you can define a function once and call it whenever needed.
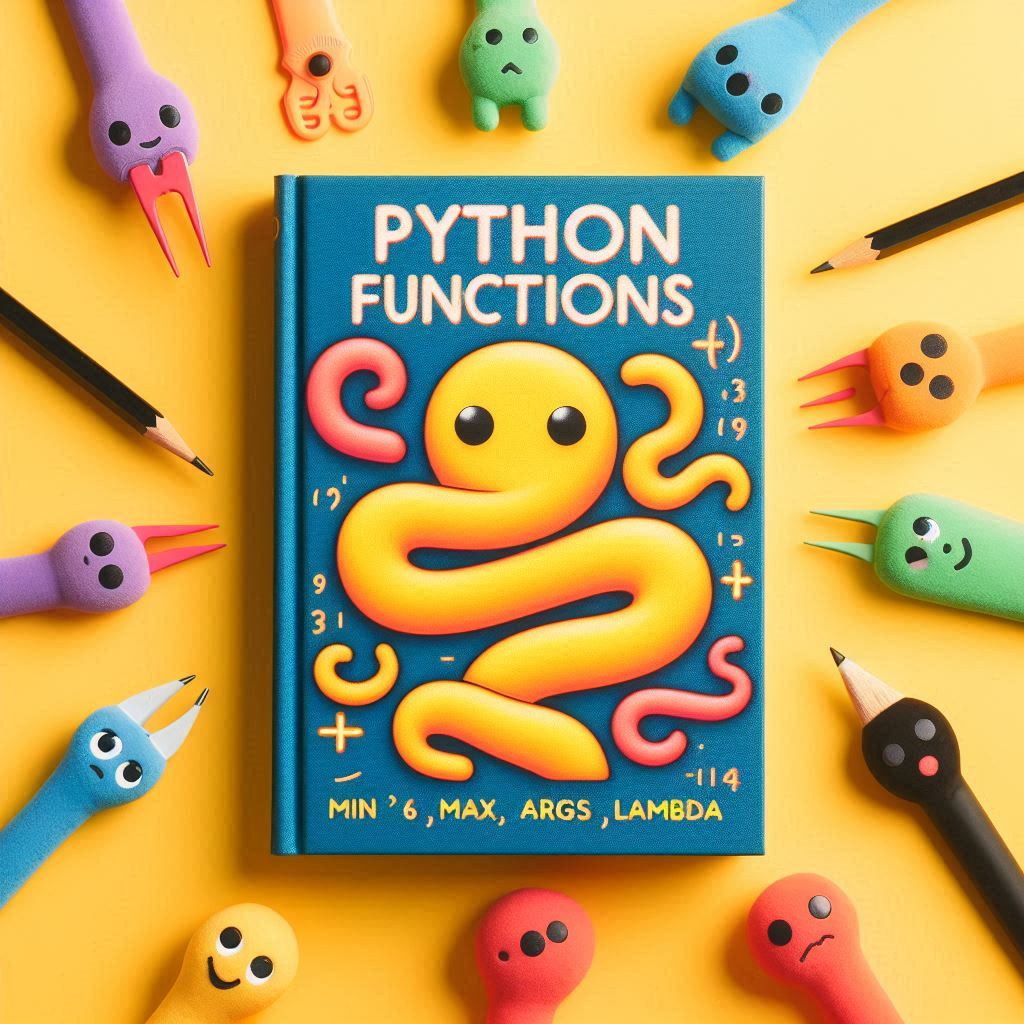
Benefits of Using Functions:
- Reusability: Write code once, and use it many times.
- Modularity: Break down complex tasks into simpler, smaller tasks.
- Maintainability: Easier to update and manage your code.
- Readability: Makes your code easier to read and understand.
Defining a Function
In Python, you define a function using the def
keyword. Here is the basic syntax for defining a function:
def function_name(parameters): """ Docstring: A description of what the function does (optional). """ # Code that performs the function's task return value # The result that the function gives back (optional)
Components of the Function Syntax
def
keyword: This keyword is used to start the function definition.
Function name: This is the name you give to your function. It should be descriptive and follow these rules:
- Start with a letter or an underscore (_).
- Can contain letters, numbers, and underscores.
- Cannot be a reserved word in Python (like
def
,return
, etc.).
Parameters (Optional): These are the values you pass to the function. They act as placeholders for the values the function will use. If your function doesn’t need any parameters, you can leave the parentheses empty.
Colon (:
): This symbol indicates the start of the function’s code block.
Docstring (Optional): This is a string that describes what the function does. It is enclosed in triple quotes (""" """
).
Function body: This is the block of code that performs the function’s task. This code runs when the function is called. The function body must be indented.
return
statement (Optional): This statement is used to give back a value from the function. If there is no return
statement, the function returns None
by default.
To use a function, you need to call it by its name and provide the necessary arguments.
Example 1:
First, let’s look at an example of a simple function which is not using parameters, return
keyword, and docstring.
def greet(): print("Hello, welcome!") greet() # Output: Hello, welcome!
Output:
Hello, welcome!
Example 2:
Now, let’s create a function that adds two numbers and returns the result.
def add_numbers(a, b): """ This function takes two numbers and returns their sum. """ result = a + b return result # Calling the function sum_result = add_numbers(5, 3) # Printing the result print(sum_result) # Output: 8
In this example:
def add_numbers(a, b)
: defines a function namedadd_numbers
that takes two parameters,a
andb
.- The docstring (
""" This function takes two numbers and returns their sum. """
) describes what the function does. result = a + b
is the function body that performs the addition.return result
gives back the sum ofa
andb
.
Python Function Parameters
When you create functions in Python, you can make them more flexible by using parameters. Parameters allow you to pass information into your functions, making them more useful and reusable. There are different types of parameters you can use in Python: positional parameters, default parameters, keyword arguments, and variable-length parameters. Let’s look at each type in detail.
Positional Parameters
Positional parameters are the most common type of parameters. When you call a function with positional parameters, the arguments are matched to the parameters based on their position in the function call.
Example:
def greet(name, age): print(f"Hello {name}! You are {age} years old.") greet("Shreya", 20)
In this example, name
is matched to “Shreya” and age
is matched to 20 based on their positions in the function call.
Output:
Hello Shreya! You are 20 years old.
Default Parameters
Default parameters allow you to set default values for parameters. If you do not provide a value for a default parameter when calling the function, the default value is used.
Example:
def greet(name, age=30): print(f"Hello {name}! You are {age} years old.") greet("Rohan") # Uses the default value for age greet("Ashish", 25) # Overrides the default value for age
Output:
Hello Rohan! You are 30 years old.
Hello Ashish! You are 25 years old.
Keyword Arguments
Keyword arguments allow you to specify arguments by the parameter name, making your function calls more readable. You can mix positional and keyword arguments, but positional arguments must come before keyword arguments.
Example:
def greet(name, age=30): print(f"Hello {name}! You are {age} years old.") greet(name="Rahul") # Uses the default value for age greet(age=40, name="Maria") # Here, we specify the name parameter after the age parameter
Output:
Hello Rahul! You are 30 years old.
Hello Maria! You are 25 years old.
Variable-Length Parameters
Now what if you don’t know how many arguments you need to pass to a function? Python allows you to handle an arbitrary number of arguments using variable-length parameters. There are two types: *args
and **kwargs
.
args
lets you pass a variable number of positional arguments to a function. Inside the function, args
is a tuple containing all the positional arguments.
**kwargs
allows you to pass a variable number of keyword arguments to a function. Inside the function, kwargs
is a dictionary containing all the keyword arguments.
Example:
# Function to calculate the sum of any number of arguments def calculate_sum(*args): # Calculate the sum of all the arguments provided total = sum(args) # Print the total sum print(f"The sum is: {total}") # Call the function with arguments 1, 2, 3, 4 calculate_sum(1, 2, 3, 4) # ----------------------------------------- # Function to print information using keyword arguments def print_info(**kwargs): # Loop through the keyword arguments dictionary for key, value in kwargs.items(): # Print each key and value pair print(f"{key}: {value}") # Call the function with keyword arguments name, age, and city print_info(name="Alia", age=25, city="Mumbai")
Output:
The sum is: 10
name: Alia
age: 25
city: Mumbai
The return Keyword
The return
keyword is used within a function to send a result back to the caller. This allows functions to produce output that can be used elsewhere in your program.
It is placed at the end of a function’s body, followed by the value or values you want to return. When a return
statement is executed, the function exits immediately, and the specified value is sent back to the caller.
If no value is specified, the function returns None
by default.
Returning a Single Value
The most straightforward use of return
is to send a single value back to the caller. Here’s a simple example:
Example:
def calculate_square(number): return number ** 2 # Calling the function and storing the result result = calculate_square(5) print(result) # Output: 25
In this example, the calculate_square
function returns the square of the input number.
Returning Multiple Values
If you want your function to return multiple values, you can achieve this by separating the values with commas in the return
statement. When the function returns multiple values, Python automatically packs them into a tuple.
Example:
def get_person_details(): name = "Jordan" age = 30 country = "India" return name, age, country person_name, person_age, person_country = get_person_details() print(person_name, person_age, person_country)
Output:
Jordan 30 India
The pass Keyword
Sometimes, you may want to define a function without any statements inside it. In such cases, you can use the pass
keyword, which is a null operation and does nothing when executed. It allows you to define a placeholder function without causing any errors.
Example:
def future_function(): pass # Calling the function future_function() # This does nothing and returns None
In this example, the future_function
is defined but not yet implemented. The pass
keyword is used to indicate that there is no code to execute yet.
The lambda function
Lambda functions in Python are small, anonymous functions. Unlike regular functions that are defined using the def
keyword, lambda functions are defined using the lambda
keyword. They are often used when you need a short function for a brief period, such as inside another function or as an argument to a higher-order function.
The syntax of a lambda function is:
lambda arguments: expression
lambda
: This is the keyword used to create a lambda function.arguments
: A comma-separated list of arguments.expression
: This is a single expression executed by the lambda function. The result of this expression is returned automatically.
Here’s a brief example to illustrate the usage of lambda functions:
Example: Adding Two Numbers
addition = lambda x, y: x + y result = addition(3, 5) print(result) # Output: 8
Lambda functions are powerful for writing concise code, but they are not suitable for complex logic or functions that require multiple statements. For such cases, it’s better to use regular named functions.
Now, let’s talk about more use cases of Lambda functions.
Lambda functions can also be combined with other built-in functions like map()
, filter()
and reduce()
for powerful and concise data transformations.
Using Lambda Functions with map()
Lambda functions are often used with map()
for concise and readable code. The map()
function is a built-in function that allows you to apply a function to every item in an iterable (like a list) and return a new iterable with the results.
Example:
# List of numbers numbers = [1, 2, 3, 4, 5] # Using map() with a lambda function to add 10 to each number result = map(lambda x: x + 10, numbers) # Converting the result to a list and printing it print(list(result)) # Output: [11, 12, 13, 14, 15]
Using Lambda Functions with filter()
The filter()
function filters elements in an iterable based on a condition defined by a function.
Example:
# List of numbers numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] # Using filter() with a lambda function to keep only even numbers result = filter(lambda x: x % 2 == 0, numbers) # Converting the result to a list and printing it print(list(result)) # Output: [2, 4, 6, 8, 10]
In this example, filter(lambda x: x % 2 == 0, numbers)
filters out the odd numbers, keeping only the even ones.
Using Lambda Functions with reduce()
The reduce()
function, which is part of the functools
module, applies a function cumulatively to the items of an iterable, reducing the iterable to a single value.
Example:
from functools import reduce # List of numbers numbers = [1, 2, 3, 4, 5] # Using reduce() with a lambda function to calculate the product of all numbers result = reduce(lambda x, y: x * y, numbers) # Printing the result print(result) # Output: 120
In this example, reduce(lambda x, y: x * y, numbers)
multiplies all the numbers in the list together, resulting in the product 120.
Local scope and global scope
When we talk about “scope”, we’re referring to where variables are accessible and how long they exist. There are two main types of scope: local scope and global scope.
Local Scope
When a variable is defined within a function, it’s said to have local scope. This means it’s only accessible within that specific function. Once the function finishes executing, the local variables are destroyed, and you can’t use them anymore.
Example:
def my_function(): x = 10 # Local variable print(x) # Output: 10 my_function() print(x) # Error: NameError: name 'x' is not defined
In this example, the variable x
is defined within the my_function()
function. It has local scope, meaning it can only be accessed within the function. Trying to access x
outside the function will result in a NameError
because the variable is not defined in the global scope.
Output:
10
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
<ipython-input-19-0d700bf68682> in <module>
4
5 my_function()
----> 6 print(x) # Error: NameError: name 'x' is not defined
NameError: name 'x' is not defined
Global Scope
Variables defined outside of any function have global scope. This means they can be accessed from anywhere in the program, including inside functions. Global variables persist throughout the entire program, as long as the program is running.
Example:
x = 10 # Global variable def my_function(): print(x) my_function() print(x)
In this example, the variable x
is defined outside the function, making it a global variable. It can be accessed both inside and outside the function.
Output:
10
10
It’s possible to have a local variable with the same name as a global variable. But then, the local variable will shadow the global variable within the function. This means the function will use the local variable instead of the global one. But outside the function, the global variable remains unchanged.
Global Keyword in Python
If you want to change the value of a global variable from inside a function, you need to use the global
keyword. This tells Python that you’re referring to the global variable, not creating a new local variable with the same name. Here’s how you will do it.
Example:
x = 10 # Global variable def my_function(): global x x = 20 # Modifying global variable print(x) # Output: 20 my_function() print(x) # Output: 20
Output:
20
20
The nonlocal Keyword in Python
Variables defined inside a function have a local scope, meaning they can only be used within that function. In nested functions (functions inside other functions), the inner function can access variables from its own local scope and from the outer function’s scope, but it can’t change the outer function’s variables.
To solve this problem, we can use nonlocal
keyword.
This keyword lets the inner function modify a variable from the outer function. It tells Python to look for the variable in the nearest enclosing scope, which is the outer function in this case.
Example:
def outer_function(): x = 10 # Variable x is defined in the outer function def inner_function(): nonlocal x # This tells Python to use the x from the outer_function x = 20 # This modifies the x in the outer_function print("Inner x:", x) inner_function() print("Outer x:", x) outer_function()
Output:
Inner x: 20
Outer x: 20